WaveVR_CameraTexture¶
Contents |
Introduction¶
WaveVR_CameraTexture.cs is a C# wrapper to gain access to the preview image from the Android camera or external camera (if one is available). And WaveVR_CameraTexture.cs provides that your scene after starting the camera can maintain rendering performance in 75 fps, or your scene is pose sensitive the camera the image will be smooth without judder. You need to add permissions as shown below to access the Android camera in AndroidManifest.xml if you want to use the CameraTexture
<uses-permission android:name=”android.permission.CAMERA” />
WaveVR_CameraTexture provides several functions to get properties of the native camera included
- isStarted
returns true if the native camera is launched.
- getImageFormat
returns image format like WVR_CameraImageFormat_YUV_420 and WVR_CameraImageFormat_Grayscale, when return WVR_CameraImageFormat_Invalid means the camera has not been launched.
- getImageType
returns image type either WVR_CameraImageType_SingleEye or WVR_CameraImageType_DualEye. We suggest to check this before you decide how to display your image.
- GetCameraImageType (Not currently supported)
returns image type either WVR_CameraImageType_SingleEye or WVR_CameraImageType_DualEye. We suggest to check this before you decide how to display your image.
- getImageWidth
returns the width of the camera image. 0 means the camera has not been launched.
- GetCameraImageWidth (Not currently supported)
returns the width of the camera image. 0 means the camera has not been launched.
- getImageHeight
returns the height of the camera image. 0 means the camera has not been launched.
- GetCameraImageHeight (Not currently supported)
returns the height of the camera image. 0 means the camera has not been launched.
- getNativeTextureId
returns the native texture id of the camera texture. 0 means the camera has not been launched.
- getImageSize
returns the buffer size for raw image data. 0 means the camera has not been launched.
- isEnableSyncPose
returns whether the current enable Syncpose state
- getNativeFrameBuffer
returns the native framebuffer as a IntPtr.
- getFramePose(ref WVR_PoseState_t)
returns true and information about WVR_PoseState_t struct, when developers use enable SyncPose feature.
returns false without information about WVR_PoseState_t struct, when developers use disable SyncPose feature.
The script also provides APIs to control the native camera including
- startCamera (Please use void startCamera(bool enableSyncPose) instead.)
returns true if the camera is ready to be launched.
- startCamera(bool enableSyncPose)
Developers can decide if they need to use SyncPose feature in this time.
Disable SyncPose : call this API with “false”, after starting the camera, the fps can keep at 75, but the trade-off is that the picture will judder.
Enable SyncPose : call this API with “true”,after starting the camera, the fps will drop at 30, but it will be more smoother than in disable SyncPose situation. If your scenes with pose sensitive we recommended use enable SyncPose. Such as CameraTexture_enableSyncPose_Test_SeeThrough .
- stopCamera
calls this API to stop the native camera.
- updateTexture(uint textureId)
calls this API with texture ID, WaveVR will update the image content of the id you provided. After the texture is updated by native runtime, UpdateCameraCompletedDelegate will be called.
Moreover, WaveVR_CameraTexture provides delegates to notify a user if the camera status has been updated. You can monitor delegates to proceed with actions after the camera status changes.
- StartCameraCompletedDelegate
StartCameraCompleted(bool result) is a prototype and will be implemented by a user who wants to monitor the camera start result and add it to StartCameraCompletedDelegate, WaveVR will notify a user with results after the camera starts.
- UpdateCameraCompletedDelegate
UpdateCameraCompleted(uint nativeTextureId) is a prototype and will be implemented by a user who wants to monitor the camera update complete event. This function will be called if there is no newer data.
Resource¶
There are two different example using WaveVR_CameraTexture , if you want to maintain rendering performance in 75 fps after starting camera, you can refer CameraTexture_disableSyncPose_Test , or you prefer smooth picture without judder, you can refer CameraTexture_enableSyncPose_Test_SeeThrough .
Script WaveVR_CameraTexture.cs is located in Assets/WaveVR/Scripts
Reference scene CameraTexture_disableSyncPose_Test is located in Assets/Samples/CameraTexture_disableSyncPose_Test/Scenes (in sample.package)
Reference scene CameraTexture_enableSyncPose_Test_SeeThrough is located in Assets/Samples/CameraTexture_enableSyncPose_Test_SeeThrough/Scenes (in sample.package)
Sample script is located in Assets/Samples/CameraTexture_disableSyncPose_Test/Scripts (in sample.package)
Sample script is located in Assets/Samples/CameraTexture_enableSyncPose_Test_SeeThrough/Scripts (in sample.package)
How to Use¶
- In this sample, we use Android camera as a camera source. Add permissions to AndroidManifest.xml.

- Open the sample scene CameraTexture_disableSyncPose_Test.
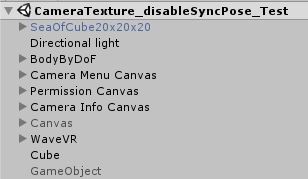
We add a cube in the hierarchy of the scene, and will update the camera image on the cube.
- Look in Inspector of CameraTexture_disableSyncPose_Test
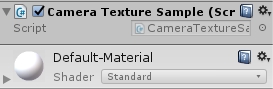
In CameraTexture_enableSyncPose_Test_SeeThrough ,we will update the camera image on SeeThroughMat material.
SeeThroughMat is located in Assets/Samples/CameraTexture_enableSyncPose_Test_SeeThrough/Materials (in sample.package)
Look in Inspector of CameraTexture_enableSyncPose_Test_SeeThrough
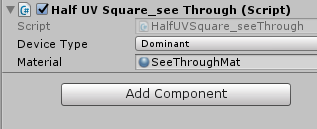
Source code¶
- Start camera
nativeTexture = new Texture2D(1280, 400);
WaveVR_CameraTexture.StartCameraCompletedDelegate += startCameraeCompleted;
WaveVR_CameraTexture.UpdateCameraCompletedDelegate += updateTextureCompleted;
WaveVR_CameraTexture.instance.startCamera(false);
textureid = nativeTexture.GetNativeTexturePtr();
meshrenderer = GetComponent<MeshRenderer>();
meshrenderer.material.mainTexture = nativeTexture;
- Implement delegates to listen to a WaveVR_CameraTexture event.
void startCameraeCompleted(bool result)
{
Log.d(LOG_TAG, "startCameraeCompleted, start? " + result);
started = result;
}
void updateTextureCompleted(uint textureId)
{
Log.d(LOG_TAG, "updateTextureCompleted, textureid = " + textureId);
meshrenderer.material.mainTexture = nativeTexture;
updated = true;
}
- Update camera texture
if (started && updated)
{
updated = false;
WaveVR_CameraTexture.instance.updateTexture((uint)textureid);
}