How Do I Show or Hide the Controller Pointer¶
Introduction¶
The VIVE Wave™ controller pointer is created by WaveVR_ControllerPointer and is shown by default. You can choose to show or hide the pointer in runtime.
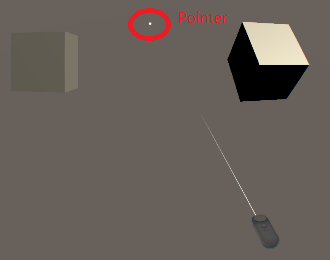
Note
- The VIVE Wave™ Event System is not affected even if the pointer is hidden.
Show or Hide The Controller Pointer¶
Follow the steps below to show or hide the controller pointer.
- Add the following code to get the controller instances.
private GameObject dominantController = null, nonDominantController = null;
void OnEnable()
{
WaveVR_Utils.Event.Listen (WaveVR_Utils.Event.CONTROLLER_MODEL_LOADED, OnControllerLoaded);
}
void OnControllerLoaded(params object[] args)
{
WaveVR_Controller.EDeviceType _type = (WaveVR_Controller.EDeviceType)args [0];
if (_type == WaveVR_Controller.EDeviceType.Dominant)
{
this.dominantController = (GameObject)args [1];
}
if (_type == WaveVR_Controller.EDeviceType.NonDominant)
{
this.nonDominantController = (GameObject)args [1];
}
}
void OnDisable()
{
WaveVR_Utils.Event.Remove (WaveVR_Utils.Event.CONTROLLER_MODEL_LOADED, OnControllerLoaded);
}
- Assume there are two functions,
ShowPointer
andHidePointer
, use the following code.
public void ShowPointer()
{
WaveVR_ControllerPointer _cp = null;
if (this.dominantController != null)
{
_cp = this.dominantController.GetComponentInChildren<WaveVR_ControllerPointer> ();
if (_cp != null)
{
_cp.ShowPointer = true;
}
}
if (this.nonDominantController != null)
{
_cp = this.nonDominantController.GetComponentInChildren<WaveVR_ControllerPointer> ();
if (_cp != null)
{
_cp.ShowPointer = true;
}
}
}
public void HidePointer()
{
WaveVR_ControllerPointer _cp = null;
if (this.dominantController != null)
{
_cp = this.dominantController.GetComponentInChildren<WaveVR_ControllerPointer> ();
if (_cp != null)
{
_cp.ShowPointer = false;
}
}
if (this.nonDominantController != null)
{
_cp = this.nonDominantController.GetComponentInChildren<WaveVR_ControllerPointer> ();
if (_cp != null)
{
_cp.ShowPointer = false;
}
}
}