WaveVR_Controller¶
Introduction¶
Note
Please refer to WaveVR API Level for API compatibility.
Note
For getting the button events, please read this document first: How to Get Button Events
WaveVR_Controller.cs provides an interface to access the controller.
By using WaveVR_Controller
, you can easily get the button state and axis as well as the pose of the controller.
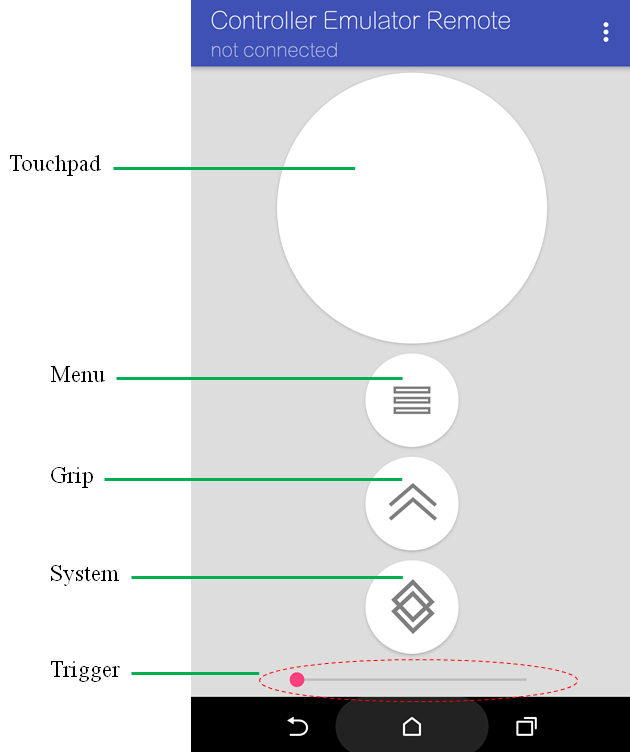
Usage¶
Device Type¶
The WaveVR API interface provides device types below:
public enum WVR_DeviceType
{
WVR_DeviceType_Invalid = 0,
WVR_DeviceType_HMD = 1,
WVR_DeviceType_Controller_Right = 2,
WVR_DeviceType_Controller_Left = 3,
};
The WaveVR Unity SDK provides device types below:
public enum EDeviceType
{
Head = 1,
Dominant = 2,
NonDominant = 3
};
Both can be used in the WaveVR_Controller, sample code:
WaveVR_Controller.Input( <device type> )
// e.g.
WaveVR_Controller.Device _dev = WaveVR_Controller.Input(WVR_DeviceType.WVR_DeviceType_Controller_Right);
// or
WaveVR_Controller.Device _dev = WaveVR_Controller.Input(WaveVR_Controller.EDeviceType.Dominant);
Right / Left means right or left hand. Please pay careful attention to the right-handed mode and the left-handed mode.
In Unity scene, an object has its own type right or left. In physics, a real device also has its own type right or left. In right-handed mode, the right device is mapping to the right Unity object. In left-handed mode, the left device is mapping to the right Unity object.
WaveVR_Controller helps you manage the type-mapping in right-handed and left-handed mode.
So, you do NOT need to worry about the real device type, just specify the Unity object type to get WaveVR_Controller.Device
.
Refer to Left-handed Mode for details.
Dominant / NonDominant means the dominant or non-dominant hand which is used to prevent right / left confusion. This is introduced in Dominant Hand
Button ID¶
The WaveVR API interface provides button IDs below:
public enum WVR_InputId
{
WVR_InputId_Alias1_System = WVR_InputId_0,
WVR_InputId_Alias1_Menu = WVR_InputId_1,
WVR_InputId_Alias1_Grip = WVR_InputId_2,
WVR_InputId_Alias1_DPad_Left = WVR_InputId_3,
WVR_InputId_Alias1_DPad_Up = WVR_InputId_4,
WVR_InputId_Alias1_DPad_Right = WVR_InputId_5,
WVR_InputId_Alias1_DPad_Down = WVR_InputId_6,
WVR_InputId_Alias1_Volume_Up = WVR_InputId_7,
WVR_InputId_Alias1_Volume_Down = WVR_InputId_8,
WVR_InputId_Alias1_Digital_Trigger = WVR_InputId_9,
WVR_InputId_Alias1_Back = WVR_InputId_14, // HMD Back Button
WVR_InputId_Alias1_Enter = WVR_InputId_15, // HMD Enter Button
WVR_InputId_Alias1_Touchpad = WVR_InputId_16,
WVR_InputId_Alias1_Trigger = WVR_InputId_17,
WVR_InputId_Alias1_Thumbstick = WVR_InputId_18,
}
The WaveVR Unity SDK provides button IDs below:
public enum EButtons
{
Unavailable = WVR_InputId.WVR_InputId_Alias1_System,
Menu = WVR_InputId.WVR_InputId_Alias1_Menu,
Grip = WVR_InputId.WVR_InputId_Alias1_Grip,
DPadUp = WVR_InputId.WVR_InputId_Alias1_DPad_Up,
DPadRight = WVR_InputId.WVR_InputId_Alias1_DPad_Right,
DPadDown = WVR_InputId.WVR_InputId_Alias1_DPad_Down,
DPadLeft = WVR_InputId.WVR_InputId_Alias1_DPad_Left,
VolumeUp = WVR_InputId.WVR_InputId_Alias1_Volume_Up,
VolumeDown = WVR_InputId.WVR_InputId_Alias1_Volume_Down,
HMDBack = WVR_InputId.WVR_InputId_Alias1_Back,
HMDEnter = WVR_InputId.WVR_InputId_Alias1_Enter,
Touchpad = WVR_InputId.WVR_InputId_Alias1_Touchpad,
Trigger = WVR_InputId.WVR_InputId_Alias1_Trigger,
Thumbstick = WVR_InputId.WVR_InputId_Alias1_Thumbstick
}
Both types are supported in WaveVR_Controller. Here is the sample code:
WaveVR_Controller.Input( <device type> ).GetPress( <Button ID> ); // whether button is pressed.
WaveVR_Controller.Input( <device type> ).GetPressDown( <Button ID> ); // whether button is pressed from unpressed, only 1 frame.
WaveVR_Controller.Input( <device type> ).GetPressUp( <Button ID> ); // whether button is released from pressed, only 1 frame.
WaveVR_Controller.Input( <device type> ).GetTouch( <Button ID> ); // whether button is touched.
WaveVR_Controller.Input( <device type> ).GetTouchDown( <Button ID> ); // whether button is touched from untouched, only 1 frame.
WaveVR_Controller.Input( <device type> ).GetTouchUp( <Button ID> ); // whether button is untouched from touched, only 1 frame.
// e.g.
WaveVR_Controller.Device _dev = WaveVR_Controller.Input(WaveVR_Controller.EDeviceType.Dominant);
bool _press = _dev.GetPress (WVR_InputId.WVR_InputId_Alias1_Touchpad); // whether touchpad of dominant hand is pressed.
Pose¶
You can access the device pose using the following sample code:
WaveVR_Controller.Input( <device type> ).transform;
// e.g.
WaveVR_Controller.Device _dev = WaveVR_Controller.Input(WaveVR_Controller.EDeviceType.Dominant);
WaveVR_Utils.RigidTransform _pose = _dev.transform; // pose of dominant hand.
Vector3 _position = _pose.pos;
Quaternion _rotation = _pose.rot;
Other Information¶
Connection: WaveVR_Controller.Device.connected
Velocity (m): WaveVR_Controller.Device.velocity
Axis of button (-1~1): WaveVR_Controller.Device.GetAxis( <Button ID> )
Vibrate device: WaveVR_Controller.Device.TriggerHapticPulse();
Left-handed Mode¶
WaveVR provides left-handed mode to users.
In typical conditions, you do NOT have to consider the left-handed mode handling in AP development.
E.g. You can use
WaveVR_Controller.Input(WVR_DeviceType.WVR_DeviceType_Controller_Right).connected
to check whether the right hand controller is connected.
In left-handed mode, this code also works and does NOT have to change.
But if you try to create different effects on a GameObject between the left / right handed mode, you will need to know which mode is currently being used.
Check the left-handed mode:
WaveVR_Controller.IsLeftHanded
Get the left-handed mode type:
WaveVR_Controller.Input ( <original type> ).DeviceType
To check the device connection:
if (WaveVR_Controller.Input(WVR_DeviceType.WVR_DeviceType_Controller_Right).connected)
{
if (WaveVR_Controller.IsLeftHanded)
{ // actually left hardware controller is connected.
// ... do something
} else
{ // actually right hardware controller is connected
// ... do something
}
}
Dominant Hand¶
The Dominant hand refers to the device that a player is accustomed to using.
E.g. In right-handed mode, the dominant hand is right. In left-handed mode, the dominant hand is left.
Thus, you can use the sample code below to get button states, device connection status and pose.
// e.g. whether trigger button is pressed down.
bool _pressed = WaveVR_Controller.Input (WaveVR_Controller.EDeviceType.Dominant).GetPressDown (WVR_InputId.WVR_InputId_Alias1_Trigger);
// e.g connection status
bool _conn = WaveVR_Controller.Input (WaveVR_Controller.EDeviceType.Dominant).connected;
// e.g. device pose
WaveVR_Utils.RigidTransform _rpose = WaveVR_Controller.Input (WaveVR_Controller.EDeviceType.Dominant).transform;
If you want to know which device is used by the dominant hand:
// get the device corresponding to dominant hand
WVR_DeviceType _dev = WaveVR_Controller.Input (WaveVR_Controller.EDeviceType.Dominant).DeviceType;