WaveVR_ControllerInputModule¶
Important: we do NOT encourage using this script directly.
Older versions (2.0.95 and before) can be found here: WaveVR_ControllerInputModule
Introduction¶
Unity has Event System which lets objects receive events from an input and take corresponding actions.
An Input Module is where the main logic of how you want the Event System to behave lives, they are used for:
- Handling input
- Managing event mechanism
- Sending events to scene objects.
This script supports an input module of multiple controllers.
Resources¶
Script WaveVR_ControllerInputModule is located in Assets/WaveVR/Scripts
Script WaveVR_EventHandler and other EventSystem scripts are located in Assets/WaveVR/Extra/EventSystem
Script GOEventTrigger is located in Assets/WaveVR/Extra
Sample ControllerInputModule_Test is located in Assets/Samples/ControllerInputModule_Test/Scenes
Prefab ControllerLoader_R is the prefab from Assets/WaveVR/Prefabs
Sample¶
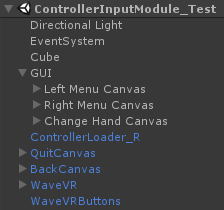
This sample demonstrates WaveVR_ControllerInputModule.cs usage.
- ControllerLoader_R
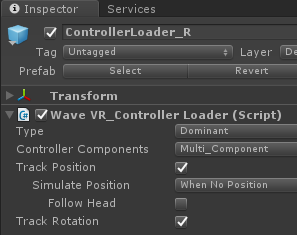
WaveVR provides the ControllerLoader prefab in Assets/WaveVR/Prefabs for loading the controller model.
In this sample, we rename the ControllerLoader to ControllerLoader_R and set the value according to the photo above.
For detailed information about ControllerLoader, please refer to WaveVR_ControllerLoader
- EventSystem
WaveVR_ControllerInputModule must be added into EventSystem like the photo below:
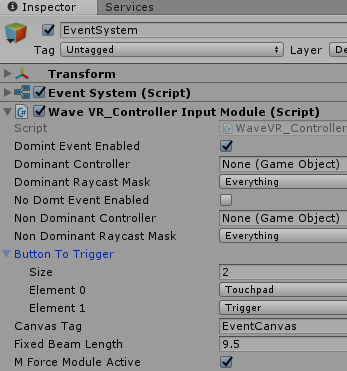
If you have a private controller model, it must be dragged into the field Right Controller
or Left Controller
.
Note: For using a private controller model, you must add the component **WaveVR_SetAsEventSystemController to mention that this controller is an Event Controller.**
E.g.
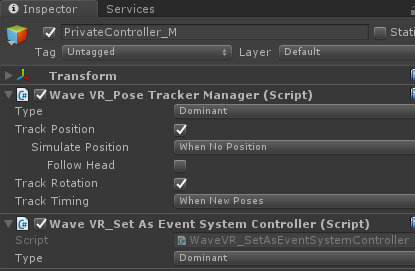
Otherwise, you can use the ControllerLoader and do NOT need to update any field of WaveVR_ControllerInputModule.
- Left Menu Canvas and Right Menu Canvas
WaveVR provides two methods for GUI being able to receive events from the Unity EventSystem.
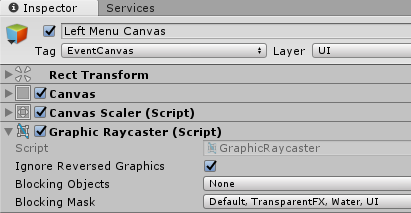
In Left Menu Canvas, the Tag
with value EventCanvas is used to mark GUI being able to receive events from EventSystem.
Note: if you use the Tag
, its value must be equivalent to the Canvas Tag
of WaveVR_ControllerInputModule.
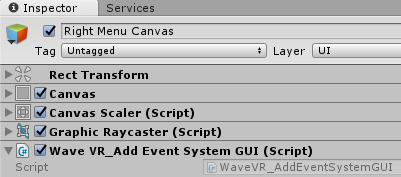
In Right Menu Canvas, the WaveVR_AddEventSystemGUI
is used to mark GUI being able to receive events from EventSystem.
Script¶
Work Flow¶
A raycaster is needed to send events. Unity uses the GraphicRaycaster
for GUI and the PhysicsRaycaster
for physical objects.
When an object is casted by the raycaster, the object will receive the Enter
event and the previously casted object will receive the Exit
event.
If the raycaster is hovering on an object, the object will receive the Hover
events continuously.
// 1. Get graphic raycast object.
ResetPointerEventData (_dt);
GraphicRaycast (_eventController, _event_camera);
if (GetRaycastedObject (_dt) == null)
{
// 2. Get physic raycast object.
PhysicsRaycaster _raycaster = _controller.GetComponent<PhysicsRaycaster> ();
if (_raycaster == null)
continue;
ResetPointerEventData (_dt);
PhysicRaycast (_eventController, _raycaster);
}
// 3. Exit previous object, enter new object.
OnTriggerEnterAndExit (_dt, _eventController.event_data);
// 4. Hover object.
GameObject _curRaycastedObject = GetRaycastedObject (_dt);
if (_curRaycastedObject != null && _curRaycastedObject == _eventController.prevRaycastedObject)
{
OnTriggerHover (_dt, _eventController.event_data);
}
When the device key is released and the casted object is a Button, the onClick
function of the Button will be invoked.
if (btnPressDown)
_eventController.eligibleForButtonClick = true;
if (btnPressUp && _eventController.eligibleForButtonClick)
onButtonClick (_eventController);
Change Event Camera When Graphic Raycast¶
The Canvas of a GUI has only one Event Camera for handling the UI events.
But you may have multiple controllers and they all trigger events to Canvas.
In order to make sure the Canvas can handle events from cameras of both controllers, you will need to switch the event camera before casting.
Considering that a scene has multiple Canvases, some have to receive events and others do not.
You only have to change the event cameras of Canvases to those that have to receive events.
For a fast way to use Tag, we provide a text field Canvas Tag in the script.
Then, you can easily get the Canvases:
GameObject[] _tag_GUIs = GameObject.FindGameObjectsWithTag (CanvasTag);
You can get the new event camera from a specified controller:
Camera _event_camera = (Camera)_controller.GetComponent (typeof(Camera));
Then, the event camera can be changed easily:
_canvas.worldCamera = event_camera;
Pointer Event Flow¶
Except for the Enter
and Exit
event of Event Trigger Type , the flow of other events is:
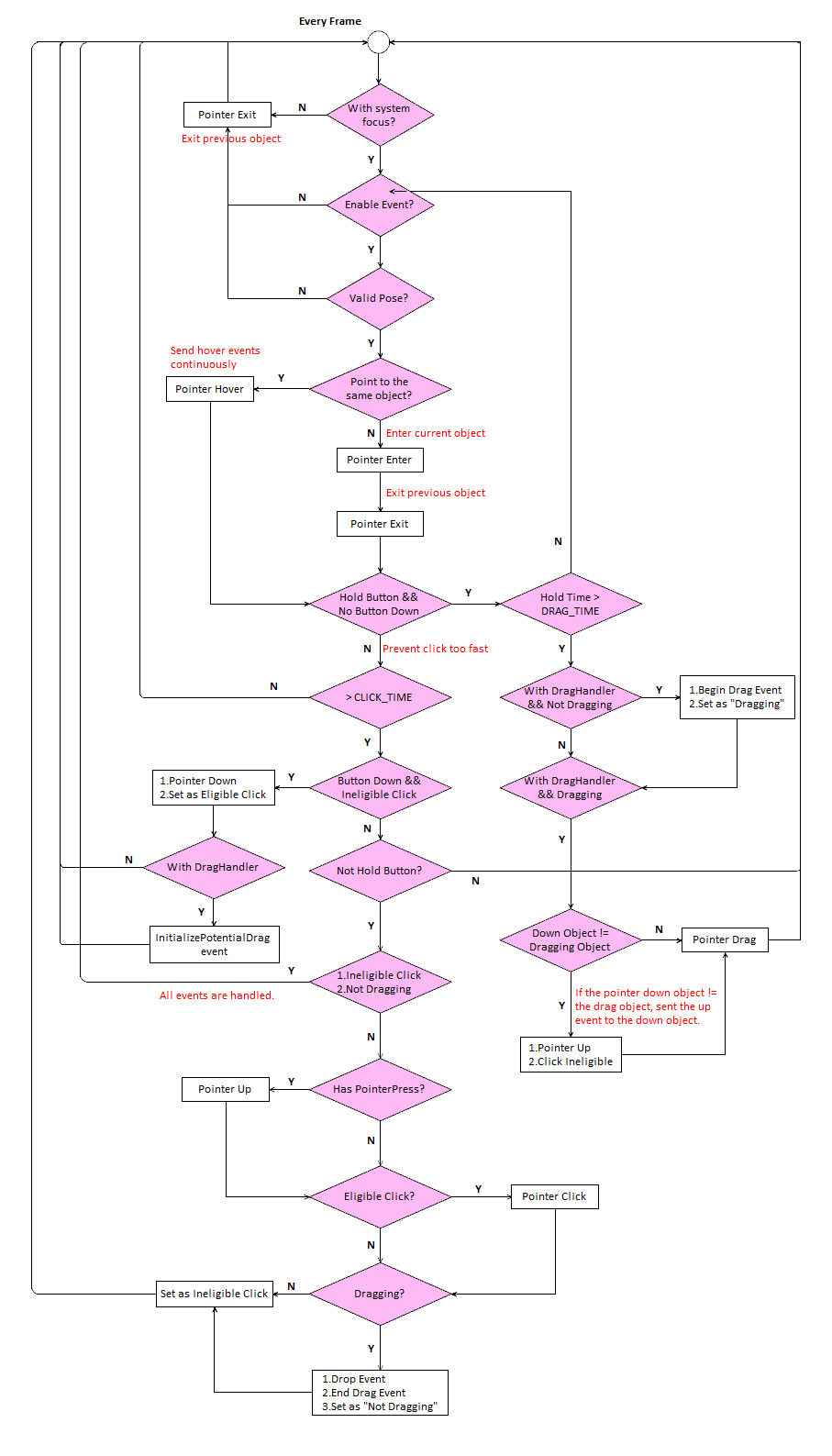
Summary:
- When the frame of a button changes briefly from unpressed to pressed:
- The Pointer Down event is sent.
- The initializePotentialDrag event is sent to the object that has a IDragHandler .
- When the frames of a button are pressed:
- The beginDrag event is sent.
- The Up event is sent to another object (different with current object) that received the Pointer Down event.
- The Drag event is sent continuously.
- When the frame of a button changes briefly from pressed to unpressed:
- The Pointer Up event is sent.
- The Pointer Click event is sent.
- If the Drag event has been sent before, the Pointer Drop event and the endDrag event will be sent.
As for the Enter
, Exit
and Hover
events, these events are not related to the button state so they have not been included in above flow:
- When raycasting an object, the
Enter
event will be sent. - When hovering over a raycasting object, the
Hover
event will be sent. - When leaving a raycating object, the
Exit
event will be sent.
Custom Defined Events¶
WaveVR defined custom EventSystem is located in Assets/WaveVR/Scripts/EventSystem
WaveVR_ExecuteEvents
is used for sending events.
IWaveVR_EventSystem
is used for receiving events.
You do not need to implement the part of sending events, as it is managed in this script.
But, if you want GameObjects with custom scripts receiving events, the scripts should inherit the corresponding event interface:
public class WaveVR_EventHandler: MonoBehaviour,
IPointerEnterHandler,
IPointerExitHandler,
IPointerDownHandler,
IBeginDragHandler,
IDragHandler,
IEndDragHandler,
IDropHandler,
IPointerHoverHandler
IPointerHoverHandler
is a WaveVR defined event which is not part of Event Trigger Type .