WaveVR_DynamicResolution¶
Introduction¶
WaveVR_DynamicResolution
is a feature which helps adjust the image quality of the application according to events sent by AdaptiveQuality. Hence, the AdaptiveQuality feature must be enabled in order to use WaveVR_DynamicResolution
and adjustments to image quality are made by changing the ResolutionScale according to the event type received from AdaptiveQuality.
When the event type received is WVR_EventType_RecommendedQuality_Higher
, the resolution will be increased.
When the event type received is WVR_EventType_RecommendedQuality_Lower
, the resolution will be decreased.
In order to ensure that the ResolutionScale will not decrease to a point where the application is unusable, WaveVR_DynamicResolution
also have another built-in functionality which helps determine the lower bound of ResolutionScale for different VR devices.
Starting from WaveVR 3.2.0, WaveVR_DynamicResolution
will be attached to the WaveVRAdaptiveQuality GameObject in the WaveVR Prefab. By default, WaveVR_DynamicResolution
is disabled, enable it manually if necessary.
Resolution Scale¶
WaveVR_Render
prepares the RenderTexture for each eye. While you can adjust the size of the RenderTexture, its aspect ratio will be kept constant.
When a ResolutionScale value is set to WaveVR_Render
, WaveVR_Render
will change the size of the RenderTexture accordingly. The new RenderTexture size is the original size multiplied by the ResolutionScale value.
The original size here refers to the size of the RenderTexture of one eye which you defined in WaveVR_Render
.
ResolutionScale has maximum value of 1
and minimum value of 0.1
, but the lowest ResolutionScale reachable during runtime is defined as either the lowest ResolutionScale value in the Resolution Scale List
or the lower bound calculated by WaveVR_DynamicResolution
, whichever is higher gets chosen.
Lower Bound of Resolution Scale¶
WaveVR_DynamicResolution
calculates a lower bound for the ResolutionScale with readability of UI text elements in mind. To put it in a simple way, as the text size gets smaller, the lower bound for the ResolutionScale increases, as more rendered pixels (i.e. larger RenderTexture size) is needed to display the text in a readable manner. The Text Size
in WaveVR_DynamicResolution
is measured in Distance-Independent Millimeters(dmm), where dmm is an angular unit. For example, 1 mm at 1 meter distance and 2 mm at 2 meter distance are both considered as 1 dmm.
To obtain the corresponding dmm from Unity UI Font Size, we must first convert Unity UI Font Size to real life units. At transform scale (1,1,1), Text height at Font Size 14 is 10 meters, so the coefficient to transform real World Space Height to Unity UI Font Size is 1.4. Since dmm uses millimeters for text height, we then convert the real World Space Height from meters to millimeters (multiply by 1000). Finally, we take the distance between the user (position of HMD) and text (Canvas for holding the UI text elements) and divide the text height with it. Hence, the conversion method from Unity UI Text Font size to dmm is as follow:
Text Size in dmm = ((Unity Font Size * Transform Scale)/ 1.4) * 1000 / Intended Reading Distance
For example, if Unity Font Size is 30, Transform Scale of the text canvas is 0.001 and you plan to make the text readable when the user is 1 meter away from the text canvas, then the Text Size should be ((30 * 0.001)/1.4) * 1000 / 1 = 21.42857~dmm. For simplicity, roundup the value to 22 dmm and set it in the inspector.
Recommended Practice: Use text of size at least 20 dmm or larger. Text of size 20 dmm - 40 dmm are commonly used in other mediums such as webpages and are known to provide a relatively comfortable reading experience. While you can always set a lower bound manually in the list which is high enough to make text of size smaller than 20 dmm readable, we do not recommend this from an application design standpoint.
See also: WaveVR_Render, WVR_EnableAdaptiveQuality , WaveVR_AdaptiveQuality , AdaptiveQuality.
Inspector¶
Text Size
int
You can set the text size according to your application design. The default text size is 20 dmm, which is also the minimum recommended text size.
Resolution Scale List
List<float>
You need to define a list of ResolutionScale for WaveVR_DynamicResolution to make adjustments accordingly. In the list, value of index 0 maps to the highest quality level, and the highest ResolutionScale value should be set here. Values of increasing index should map to lower quality levels, and lower ResolutionScale values should be set in decreasing numerical order.
When
WaveVR_DynamicResolution
receives events of typeWVR_EventType_RecommendedQuality_Higher
, it will decrease the current index by 1 (one quality level higher) and take the ResolutionScale value with the new index from the list and set it toWaveVR_Render
.When
WaveVR_DynamicResolution
receives events of typeWVR_EventType_RecommendedQuality_Lower
, it will increase the current index by 1 (one quality level lower) and take the ResolutionScale value with the new index from the list and set it toWaveVR_Render
.Default Index
int
The ResolutionScale value at the Default Index in the list be the the initial value set to
WaveVR_Render
before receiving any events from AdaptiveQuality.
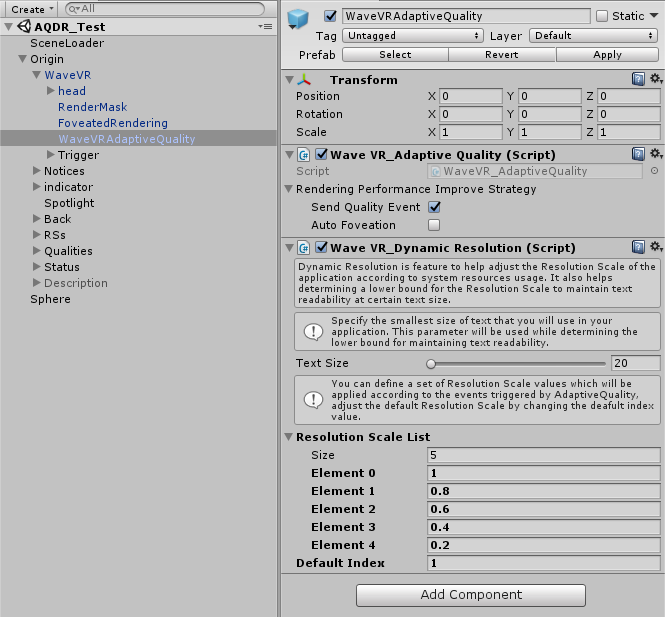
WaveVRAdaptiveQuality GameObject in WaveVR Prefab
Script¶
Public Types¶
enum AQEvent {
None,
ManualHigher,
ManualLower,
Higher,
Lower
};
None
means no events are received. ManualHigher
and ManualLower
means that the change in ResolutionScale is triggered by using public member function manually. Higher
and Lower
means that the received event was sent by AdaptiveQuality.
Properties¶
float CurrentScale { get; }
Get the mapped ResolutionScale value of the current index from the Resolution Scale List
.
AQEvent CurrentAQEvent { get; }
Get the current received event sent by AdaptiveQuality or manual trigger.
Public Member Functions¶
void Higher()
Manually increase the quality instead of waiting for an event from AdaptiveQuality. It will decrease current index by 1.
void Lower()
Manually reduce the quality instead of waiting for an event from AdaptiveQuality. It will increase current index by 1.
void Reset()
Reset the current index to default index.