Hand¶
Introduction¶
VIVE Wave™ plugin provides Blueprint APIs for miscellaneous information of Hand Tracking.
Besides the Blueprint APIs, VIVE Wave™ plugin also provides UActorComponents . For more information, please refer to Hand ActorComponent.
Public Types¶
VIVE Wave™ plugin provides Hand Tracking and Hand Gesture Blueprint types listed below.
EWaveVRTrackerType¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRTrackerType : uint8
{
Invalid = 0,
Natural = 1,//WVR_HandTrackerType::WVR_HandTrackerType_Natural,
Electronic = 2,//WVR_HandTrackerType::WVR_HandTrackerType_Electronic,
};
EWaveVRHandTrackingStatus¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRHandTrackingStatus : uint8
{
// Initial, can call Start API in this state.
NOT_START,
START_FAILURE,
// Processing, should NOT call API in this state.
STARTING,
STOPING,
// Running, can call Stop API in this state.
AVAILABLE,
// Do nothing.
UNSUPPORT
};
You can refer to the state machine diagram below for a better understanding of the Hand Tracking lifecycle:
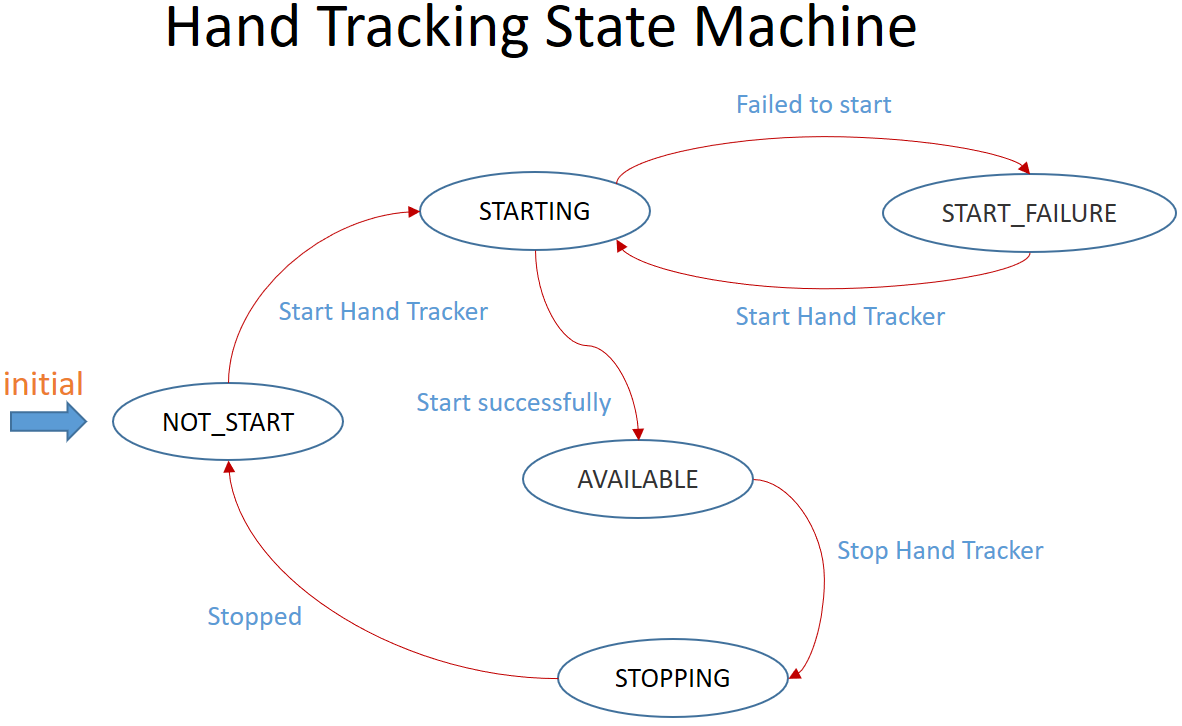
EWaveVRHandType¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRHandType : uint8
{
Left,
Right
};
EWaveVRHandMotion¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRHandMotion : uint8
{
Invalid = 0,//WVR_HandPoseType::WVR_HandPoseType_Invalid,
Pinch = 1,//WVR_HandPoseType::WVR_HandPoseType_Pinch,
Hold = 2,//WVR_HandPoseType::WVR_HandPoseType_Hold
};
EWaveVRHandJoint¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRHandJoint : uint8
{
Palm = 0,//WVR_HandJoint::WVR_HandJoint_Palm,
Wrist = 1,//WVR_HandJoint::WVR_HandJoint_Wrist,
Thumb_Joint0 = 2,//WVR_HandJoint::WVR_HandJoint_Thumb_Joint0,
Thumb_Joint1 = 3,//WVR_HandJoint::WVR_HandJoint_Thumb_Joint1,
Thumb_Joint2 = 4,//WVR_HandJoint::WVR_HandJoint_Thumb_Joint2, // 5
Thumb_Tip = 5,//WVR_HandJoint::WVR_HandJoint_Thumb_Tip,
Index_Joint0 = 6,//WVR_HandJoint::WVR_HandJoint_Index_Joint0,
Index_Joint1 = 7,//WVR_HandJoint::WVR_HandJoint_Index_Joint1,
Index_Joint2 = 8,//WVR_HandJoint::WVR_HandJoint_Index_Joint2,
Index_Joint3 = 9,//WVR_HandJoint::WVR_HandJoint_Index_Joint3, // 10
Index_Tip = 10,//WVR_HandJoint::WVR_HandJoint_Index_Tip,
Middle_Joint0 = 11,//WVR_HandJoint::WVR_HandJoint_Middle_Joint0,
Middle_Joint1 = 12,//WVR_HandJoint::WVR_HandJoint_Middle_Joint1,
Middle_Joint2 = 13,//WVR_HandJoint::WVR_HandJoint_Middle_Joint2,
Middle_Joint3 = 14,//WVR_HandJoint::WVR_HandJoint_Middle_Joint3, // 15
Middle_Tip = 15,//WVR_HandJoint::WVR_HandJoint_Middle_Tip,
Ring_Joint0 = 16,//WVR_HandJoint::WVR_HandJoint_Ring_Joint0,
Ring_Joint1 = 17,//WVR_HandJoint::WVR_HandJoint_Ring_Joint1,
Ring_Joint2 = 18,//WVR_HandJoint::WVR_HandJoint_Ring_Joint2,
Ring_Joint3 = 19,//WVR_HandJoint::WVR_HandJoint_Ring_Joint3, // 20
Ring_Tip = 20,//WVR_HandJoint::WVR_HandJoint_Ring_Tip,
Pinky_Joint0 = 21,//WVR_HandJoint::WVR_HandJoint_Pinky_Joint0,
Pinky_Joint1 = 22,//WVR_HandJoint::WVR_HandJoint_Pinky_Joint1,
Pinky_Joint2 = 23,//WVR_HandJoint::WVR_HandJoint_Pinky_Joint2,
Pinky_Joint3 = 24,//WVR_HandJoint::WVR_HandJoint_Pinky_Joint3, // 25
Pinky_Tip = 25,//WVR_HandJoint::WVR_HandJoint_Pinky_Tip,
};
VIVE Wave™ plugin defines hand joints as below photo.
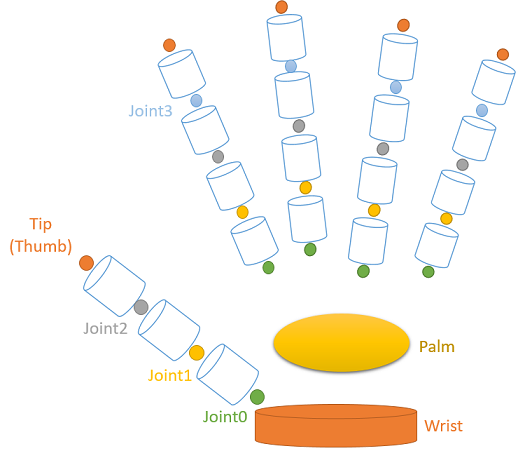
EWaveVRHandHoldObjectType¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRHandHoldObjectType : uint8
{
None = 0, //WVR_HandHoldObjectType::WVR_HandHoldObjectType_None
Gun = 1, //WVR_HandHoldObjectType::WVR_HandHoldObjectType_Gun
OCSpray = 2, //WVR_HandHoldObjectType::WVR_HandHoldObjectType_OCSpray
LongGun = 3, //WVR_HandHoldObjectType::WVR_HandHoldObjectType_LongGun
Baton = 4, //WVR_HandHoldObjectType::WVR_HandHoldObjectType_Baton
FlashLight = 5, //WVR_HandHoldObjectType::WVR_HandHoldObjectType_FlashLight
};
EWaveVRHandHoldRoleType¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRHandHoldRoleType : uint8
{
None = 0, //WVR_HandHoldRoleType::WVR_HandHoldRoleType_None
MainHold = 1, //WVR_HandHoldRoleType::WVR_HandHoldRoleType_MainHold
SideHold = 2, //WVR_HandHoldRoleType::WVR_HandHoldRoleType_SideHold
};
EWaveVRFingerType¶
enum class EWaveVRFingerType : uint8
{
None = 0,
Thumb = 1, //WVR_FingerType::WVR_FingerType_Thumb
Index = 2, //WVR_FingerType::WVR_FingerType_Index
Middle = 3, //WVR_FingerType::WVR_FingerType_Middle
Ring = 4, //WVR_FingerType::WVR_FingerType_Ring
Pinky = 5, //WVR_FingerType::WVR_FingerType_Pinky
};
EWaveVRHandGestureStatus¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRHandGestureStatus : uint8
{
// Initial, can call Start API in this state.
NOT_START,
START_FAILURE,
// Processing, should NOT call API in this state.
STARTING,
STOPING,
// Running, can call Stop API in this state.
AVAILABLE,
// Do nothing.
UNSUPPORT
};
You can refer to the state machine diagram below for a better understanding of the Hand Gesture lifecycle:
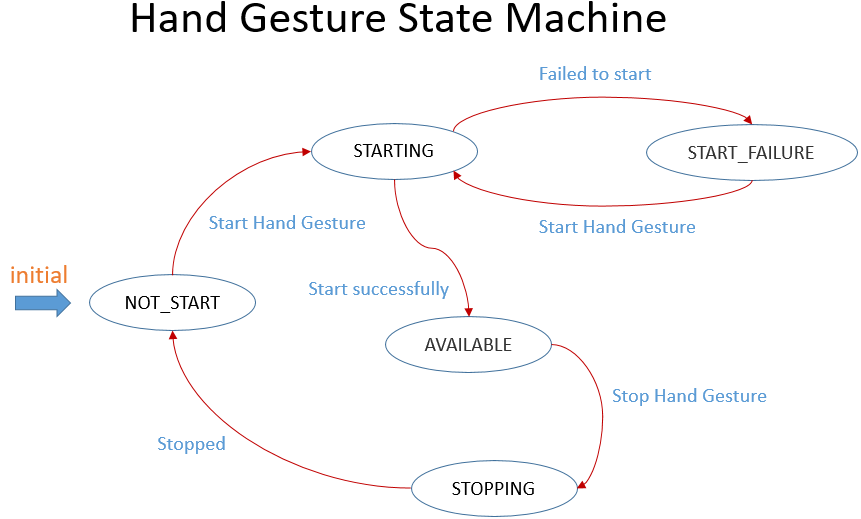
EWaveVRGestureType¶
Note
Which gesture types are supported depends on the system version.
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRGestureType : uint8
{
Invalid = 0,//WVR_HandGestureType::WVR_HandGestureType_Invalid, /**< The gesture is invalid. */
Unknown = 1,//WVR_HandGestureType::WVR_HandGestureType_Unknown, /**< Unknown gesture type. */
Fist = 2,//WVR_HandGestureType::WVR_HandGestureType_Fist, /**< Represent fist gesture. */
Five = 3,//WVR_HandGestureType::WVR_HandGestureType_Five, /**< Represent five gesture. */
OK = 4,//WVR_HandGestureType::WVR_HandGestureType_OK, /**< Represent ok gesture. */
Like = 5,//WVR_HandGestureType::WVR_HandGestureType_ThumbUp, /**< Represent thumb up gesture. */
Point = 6,//WVR_HandGestureType::WVR_HandGestureType_IndexUp, /**< Represent index up gesture. */
Palm_Pinch = 7,//WVR_HandGestureType::WVR_HandGestureType_Palm_Pinch,
Yeah = 8,//WVR_HandGestureType::WVR_HandGestureType_Yeah /**< Represent yeah gesture. */
};
Public Member Functions¶
VIVE Wave™ plugin provides Hand Tracking and Hand Gesture Blueprint API listed below.
StartHandTracking¶
Starts Hand Tracking of a specified tracker.
Note
Only the natural hand tracker is supported currently.
void StartHandTracking(EWaveVRTrackerType tracker)
StopHandTracking¶
Stops Hand Tracking of a specified tracker.
void StopHandTracking(EWaveVRTrackerType tracker)
IsHandTrackingAvailable¶
To check if Hand Tracking is available currently.
bool IsHandTrackingAvailable(EWaveVRTrackerType tracker)
GetHandTrackingStatus¶
Retrieves current Hand Tracking status.
EWaveVRHandTrackingStatus GetHandTrackingStatus(EWaveVRTrackerType tracker)
IsHandPoseValid¶
To check if current hand pose is valid.
bool IsHandPoseValid(EWaveVRTrackerType tracker, EWaveVRHandType hand)
GetHandJointPose¶
Retrieves poses of a left/right hand joint.
bool GetHandJointPose(EWaveVRTrackerType tracker, EWaveVRHandType hand, EWaveVRHandJoint joint, FVector& OutPosition, FRotator& OutRotation)
GetAllHandJointPoses¶
Retrieves poses of all left/right hand joints.
bool GetAllHandJointPoses(EWaveVRTrackerType tracker, EWaveVRHandType hand, TArray<FVector>& OutPositions, TArray<FQuat>& OutRotations)
GetHandConfidence¶
Retrieves the hand confidence (0~1), 1 means the most accurate.
float GetHandConfidence(EWaveVRTrackerType tracker, EWaveVRHandType hand)
GetHandMotion¶
Retrieves current hand motion.
EWaveVRHandMotion GetHandMotion(EWaveVRTrackerType tracker, EWaveVRHandType hand)
GetHandPinchThreshold¶
Retrieves the hand pinch on strength recommended threshold (0~1), 1 means the thumb and index finger tip are touching.
float GetHandPinchThreshold(EWaveVRTrackerType tracker)
GetHandPinchOffThreshold¶
Retrieves the hand pinch off strength recommended threshold (0~1), 1 means the thumb and index finger tip are touching.
float GetHandPinchOffThreshold(EWaveVRTrackerType tracker)
IsHandPinching¶
Checks if a hand is pinching currently in runtime.
bool IsHandPinching(EWaveVRTrackerType tracker, EWaveVRHandType hand)
GetHandPinchStrength¶
Retrieves the hand pinch strength (0~1), 1 means the thumb and index finger tip are touching. You can simply consider a hand is currently pinching when the pinch strength is bigger than the pinch threshold.
float UWaveVRHandBPLibrary::GetHandPinchStrength(EWaveVRTrackerType tracker, EWaveVRHandType hand)
As an example, here is a pinch motion visualization with a pinch strength value of 0.7f.
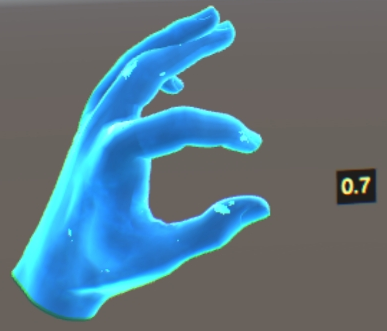
GetHandPinchOrigin¶
Retrieves the hand pinch origin in the world space.
FVector GetHandPinchOrigin(EWaveVRTrackerType tracker, EWaveVRHandType hand)
GetHandPinchDirection¶
Retrieves the hand pinch direction in the world space.
FVector GetHandPinchDirection(EWaveVRTrackerType tracker, EWaveVRHandType hand)
Refer to below photo about the pinch origin and direction.
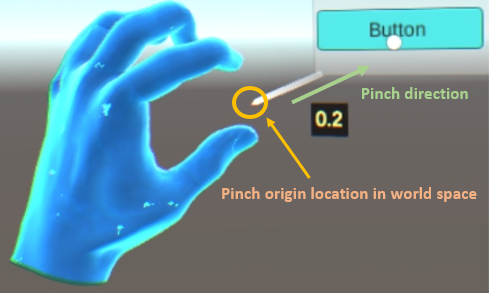
GetHandGraspStrength¶
Retrieves the hand grasp strength (0~1), 1 means the hand is grabbing like a fist.
float GetHandGraspStrength(EWaveVRTrackerType tracker, EWaveVRHandType hand)
IsHandGrasping¶
Checks if a hand is grasping currently in runtime. Note that the grasp strength is not related to this value.
bool IsHandGrasping(EWaveVRTrackerType tracker, EWaveVRHandType hand)
GetHandHoldRole¶
To retrieve the hand’s rold when holding.
EWaveVRHandHoldRoleType GetHandHoldRole(EWaveVRTrackerType tracker, EWaveVRHandType hand)
GetHandHoldType¶
To retrieve the hand hold object type when holding.
EWaveVRHandHoldObjectType GetHandHoldType(EWaveVRTrackerType tracker, EWaveVRHandType hand)
GetHandScale¶
To retrieve the hand size scale which is compared to our default model.
FVector GetHandScale(EWaveVRTrackerType tracker, EWaveVRHandType hand)
FuseWristPositionWithTracker¶
To fuse the wrist position with a wore tracker’s tracking.
void FuseWristPositionWithTracker(bool fuse)
IsWristPositionFused¶
Checks if the wrist position is fused with a wore tracker.
bool IsWristPositionFused()
ActivateHoldMotion¶
*To enable/disable the hold motion. if disabled, the hand motion will never be *
void ActivateHoldMotion(bool active)
IsHandGestureAvailable¶
To check if the Hand Gesture component is available.
bool IsHandGestureAvailable()
GetHandGestureStatus¶
To check current Hand Gesture status.
EWaveVRHandGestureStatus GetHandGestureStatus()
GetStaticGestureType¶
If the Hand Gesture component is available, this API is used to retrieve current static gesture type.
EWaveVRGestureType GetStaticGestureType(EWaveVRHandType hand)