Overview¶
VIVE Wave™ Unreal plugin version: 4.1.0
Public Types¶
EWVR_DeviceType¶
UENUM(BlueprintType)
enum class EWVR_DeviceType : uint8
{
DeviceType_Invalid = 0, //WVR_DeviceType::WVR_DeviceType_Invalid,
DeviceType_HMD = 1, //WVR_DeviceType::WVR_DeviceType_HMD,
DeviceType_Controller_Right = 2, //WVR_DeviceType::WVR_DeviceType_Controller_Right,
DeviceType_Controller_Left = 3, //WVR_DeviceType::WVR_DeviceType_Controller_Left,
DeviceType_Camera = 4, //WVR_DeviceType::WVR_DeviceType_Camera,
DeviceType_EyeTracking = 5, //WVR_DeviceType::WVR_DeviceType_EyeTracking,
DeviceType_HandGesture_Right = 6, //WVR_DeviceType::WVR_DeviceType_HandGesture_Right,
DeviceType_HandGesture_Left = 7, //WVR_DeviceType::WVR_DeviceType_HandGesture_Left,
DeviceType_NaturalHand_Right = 8, //WVR_DeviceType::WVR_DeviceType_NaturalHand_Right,
DeviceType_NaturalHand_Left = 9, //WVR_DeviceType::WVR_DeviceType_NaturalHand_Left,
DeviceType_ElectronicHand_Right = 10, //WVR_DeviceType::WVR_DeviceType_ElectronicHand_Right,
DeviceType_ElectronicHand_Left = 11, //WVR_DeviceType::WVR_DeviceType_ElectronicHand_Left,
};
EWVR_InputId¶
UENUM(BlueprintType)
enum class EWVR_InputId : uint8
{
NoUse = 0, //WVR_InputId::WVR_InputId_Alias1_System,
Menu = 1, //WVR_InputId::WVR_InputId_Alias1_Menu,
Grip = 2, //WVR_InputId::WVR_InputId_Alias1_Grip,
DPad_Left = 3, //WVR_InputId::WVR_InputId_Alias1_DPad_Left,
DPad_Up = 4, //WVR_InputId::WVR_InputId_Alias1_DPad_Up,
DPad_Right = 5, //WVR_InputId::WVR_InputId_Alias1_DPad_Right,
DPad_Down = 6, //WVR_InputId::WVR_InputId_Alias1_DPad_Down,
Volume_Up = 7, //WVR_InputId::WVR_InputId_Alias1_Volume_Up,
Volume_Down = 8, //WVR_InputId::WVR_InputId_Alias1_Volume_Down,
Bumper = 9, //WVR_InputId::WVR_InputId_Alias1_Bumper,
A = 10, //WVR_InputId::WVR_InputId_Alias1_A,
B = 11, //WVR_InputId::WVR_InputId_Alias1_B,
X = 12, //WVR_InputId::WVR_InputId_Alias1_X,
Y = 13, //WVR_InputId::WVR_InputId_Alias1_Y,
Back = 14, //WVR_InputId::WVR_InputId_Alias1_Back,
Enter = 15, //WVR_InputId::WVR_InputId_Alias1_Enter,
Touchpad = 16, //WVR_InputId::WVR_InputId_Alias1_Touchpad,
Trigger = 17, //WVR_InputId::WVR_InputId_Alias1_Trigger,
Thumbstick = 18, //WVR_InputId::WVR_InputId_Alias1_Thumbstick,
//Max = 32,
};
EWVR_TouchId¶
UENUM(BlueprintType)
enum class EWVR_TouchId : uint8
{
NoUse = 0, //WVR_InputId::WVR_InputId_Alias1_System,
Grip = 2, //WVR_InputId::WVR_InputId_Alias1_Grip,
A = 10, //WVR_InputId::WVR_InputId_Alias1_A,
B = 11, //WVR_InputId::WVR_InputId_Alias1_B,
X = 12, //WVR_InputId::WVR_InputId_Alias1_X,
Y = 13, //WVR_InputId::WVR_InputId_Alias1_Y,
Touchpad = 16, //WVR_InputId::WVR_InputId_Alias1_Touchpad,
Trigger = 17, //WVR_InputId::WVR_InputId_Alias1_Trigger,
Thumbstick = 18, //WVR_InputId::WVR_InputId_Alias1_Thumbstick,
Parking = 19, //WVR_InputId::WVR_InputId_Alias1_Parking
};
EWVR_Hand¶
UENUM(BlueprintType)
enum class EWVR_Hand : uint8
{
Hand_Controller_Invalid = 0,
Hand_Controller_Right = 2, // WVR_DeviceType::WVR_DeviceType_Controller_Right
Hand_Controller_Left = 3 // WVR_DeviceType::WVR_DeviceType_Controller_Left
};
EBeamMode¶
UENUM(BlueprintType)
enum class EBeamMode : uint8
{
Flexible = 0,
Fixed = 1,
};
EWVRInteractionMode¶
UENUM(BlueprintType)
enum class EWVRInteractionMode : uint8
{
Invalid = 0,
Default = 1, //WVR_InteractionMode::WVR_InteractionMode_SystemDefault,
Gaze = 2, //WVR_InteractionMode::WVR_InteractionMode_Gaze,
Controller = 3, //WVR_InteractionMode::WVR_InteractionMode_Controller,
Hand = 4, //WVR_InteractionMode::WVR_InteractionMode_Hand,
};
EWVRGazeTriggerType¶
UENUM(BlueprintType)
enum class EWVRGazeTriggerType : uint8
{
Invalid = 0,
Timeout = 1, //WVR_GazeTriggerType::WVR_GazeTriggerType_Timeout,
Button = 2, //WVR_GazeTriggerType::WVR_GazeTriggerType_Button,
TimeoutButton = 3, //WVR_GazeTriggerType::WVR_GazeTriggerType_TimeoutButton,
};
EWVR_DOF¶
UENUM(BlueprintType)
enum class EWVR_DOF : uint8
{
DOF_3,
DOF_6,
DOF_SYSTEM
};
SimulatePosition¶
UENUM(BlueprintType)
enum class SimulatePosition : uint8
{
WhenNoPosition = 0, // simulate when 3DoF.
ForceSimulation = 1, // force using simulation pose.
NoSimulation = 2
};
ERecenterType¶
UENUM(BlueprintType)
enum class ERecenterType: uint8
{
Disabled = 0, /**< Make everything back to system coordinate instantly */
YawOnly = 1, /**< Only adjust the Yaw angle */
YawAndPosition = 2, /**< Adjust the Yaw angle, and also reset user's position to Virtual World's center */
RotationAndPosition = 3, /**< Affect all the XYZ and Pitch Yaw Roll. It is sensitive to user's head gesture at recetnering. */
Position = 4 /**< Reset user's position to Virtual world's center */
};
EUTex2DFmtType¶
UENUM(BlueprintType)
enum class EUTex2DFmtType : uint8
{
BMP = 0, /** Note: BMP Image format */
PNG = 1, /** Note: PNG Image format */
JPEG = 2 /** Note: JPEG Image format */
};
EWVR_FoveationMode¶
UENUM(BlueprintType)
enum class EWVR_FoveationMode: uint8
{
Disable = 0, /**< **WVR_FoveationMode_Disable**: Disable foveated rendering. */
Enable = 1, /**< **WVR_FoveationMode_Enable**: Enable foveated rendering and using @ref WVR_SetFoveationConfig to set config. */
Default = 2, /**< **WVR_FoveationMode_Default**: Default config depending on the device. Using @ref WVR_IsRenderFoveationDefaultOn and @ref WVR_GetFoveationDefaultConfig to query details. */
Dynamic = 3, /**< **WVR_FoveationMode_Dynamic**: Similar to default mode, but config will dynamically change due to HMD motion. */
};
EWVR_PeripheralQuality¶
UENUM(BlueprintType)
enum class EWVR_PeripheralQuality : uint8
{
Low = 0, /**< **WVR_PeripheralQuality_High**: Peripheral quity is high and power saving is low. */
Medium = 1, /**< **WVR_PeripheralQuality_Medium**: Peripheral quity is medium and power saving is medium. */
High = 2 /**< **WVR_PeripheralQuality_Low**: Peripheral quity is low and power saving is high. */
};
EEye¶
UENUM(BlueprintType)
enum class EEye : uint8
{
LEFT = 0,
RIGHT = 1
};
EScreenshotMode¶
UENUM(BlueprintType)
enum class EScreenshotMode : uint8
{
DEFAULT = 0,
RAW,
DISTORTED
};
ERenderMaskMode¶
UENUM(BlueprintType)
enum class ERenderMaskMode : uint8
{
DISABLE = 0, /* Render mask disable */
ENABLE = 1, /* Render mask enable */
DEFAULT = 2 /* Render mask follow the global setting to enable or disable */
};
EAdaptiveQualityMode¶
UENUM(BlueprintType)
enum class EAdaptiveQualityMode : uint8
{
Quality_Oriented = 0, /* SendQualityEvent On, AutoFoveation On. */
Performance_Oriented = 1, /* SendQualityEvent On, AutoFoveation On. */
Customization = 2 /* Choose SendQualityEvent and AutoFoveation manually. */
};
EAMCMode¶
UENUM(BlueprintType)
enum class EAMCMode : uint8
{
Off, /* Always Off. */
Force_UMC, /* Use UMC. */
Auto, /* UMC will dynamicly turn on or off due to rendering and performance status. */
//Force_PMC /* Use PMC. */
};
EAMCStatus¶
UENUM(BlueprintType)
enum class EAMCStatus : uint8
{
Off, /* Off. */
UMC, /* Use UMC. */
//PMC /* Use PMC. */
};
EWVR_ControllerPoseMode¶
UENUM(BlueprintType)
enum class EWVR_ControllerPoseMode : uint8
{
ControllerPoseMode_Raw = 0, /**< Raw mode (default). It would be the same as one of three other modes (Trigger/Panel/Handle). */
ControllerPoseMode_Trigger = 1, /**< Trigger mode: Controller ray is parallel to the trigger button of controller. */
ControllerPoseMode_Panel = 2, /**< Panel mode: Controller ray is parallel to the panel of controller. */
ControllerPoseMode_Handle = 3, /**< Handle mode: Controller ray is parallel to the handle of controller. */
};
EWVR_CoordinateSystem¶
UENUM(BlueprintType)
enum class EWVR_CoordinateSystem : uint8
{
/**< The tracking data is based on local coordinate system. */
Local = 0, //WVR_CoordinateSystem_Local
/**< The tracking data is based on global coordinate system. */
World = 1, //WVR_CoordinateSystem_Global
};
EWVR_DeviceErrorStatus¶
UENUM(BlueprintType, Category = "WaveVR", meta = (DisplayName = "DeviceErrorStatus"))
enum class EWVR_DeviceErrorStatus : uint8
{
/* Status of the device service is normal. */
None = 0,
/* Battery temperature is too hot. */
BatteryOverheat = 1,
/* Battery temperature has cooled down. */
BatteryOverheatRestore = 2,
/* Battery is overvoltage when charging. */
BatteryOvervoltage = 3,
/* Overvoltage has been fixed. */
BatteryOvervoltageRestore = 4,
/* Failed to connect to the USB device. */
DeviceConnectFail = 5,
/* Connection failure has been fixed. */
DeviceConnectRestore = 6,
/* The device being tracked might be out of range. */
DeviceLostTracking = 7,
/* Lost tracking has been fixed. */
DeviceTrackingRestore = 8,
/* The consumed voltage is larger than the charging voltage when the device is charging. */
ChargeFail = 9,
/* Charging failure has been fixed. */
ChargeRestore = 10,
};
EWaveVRHandType¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRHandType : uint8
{
Left,
Right
};
EWaveVRGestureType¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRGestureType : uint8
{
Invalid = 0,//WVR_HandGestureType::WVR_HandGestureType_Invalid, /**< The gesture is invalid. */
Unknown = 1,//WVR_HandGestureType::WVR_HandGestureType_Unknown, /**< Unknown gesture type. */
Fist = 2,//WVR_HandGestureType::WVR_HandGestureType_Fist, /**< Represent fist gesture. */
Five = 3,//WVR_HandGestureType::WVR_HandGestureType_Five, /**< Represent five gesture. */
OK = 4,//WVR_HandGestureType::WVR_HandGestureType_OK, /**< Represent ok gesture. */
Like = 5,//WVR_HandGestureType::WVR_HandGestureType_ThumbUp, /**< Represent thumb up gesture. */
Point = 6,//WVR_HandGestureType::WVR_HandGestureType_IndexUp, /**< Represent index up gesture. */
Inverse = 7,//WVR_HandGestureType::WVR_HandGestureType_Inverse,
};
EWaveVRTrackerType¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRTrackerType : uint8
{
Invalid = 0,
Natural = 1,//WVR_HandTrackerType::WVR_HandTrackerType_Natural,
Electronic = 2,//WVR_HandTrackerType::WVR_HandTrackerType_Electronic,
};
EWaveVRHandMotion¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRHandMotion : uint8
{
Invalid = 0,//WVR_HandPoseType::WVR_HandPoseType_Invalid,
Pinch = 1,//WVR_HandPoseType::WVR_HandPoseType_Pinch,
};
EWaveVRHandJoint¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRHandJoint : uint8
{
Palm = 0,//WVR_HandJoint::WVR_HandJoint_Palm,
Wrist = 1,//WVR_HandJoint::WVR_HandJoint_Wrist,
Thumb_Joint0 = 2,//WVR_HandJoint::WVR_HandJoint_Thumb_Joint0,
Thumb_Joint1 = 3,//WVR_HandJoint::WVR_HandJoint_Thumb_Joint1,
Thumb_Joint2 = 4,//WVR_HandJoint::WVR_HandJoint_Thumb_Joint2, // 5
Thumb_Tip = 5,//WVR_HandJoint::WVR_HandJoint_Thumb_Tip,
Index_Joint0 = 6,//WVR_HandJoint::WVR_HandJoint_Index_Joint0,
Index_Joint1 = 7,//WVR_HandJoint::WVR_HandJoint_Index_Joint1,
Index_Joint2 = 8,//WVR_HandJoint::WVR_HandJoint_Index_Joint2,
Index_Joint3 = 9,//WVR_HandJoint::WVR_HandJoint_Index_Joint3, // 10
Index_Tip = 10,//WVR_HandJoint::WVR_HandJoint_Index_Tip,
Middle_Joint0 = 11,//WVR_HandJoint::WVR_HandJoint_Middle_Joint0,
Middle_Joint1 = 12,//WVR_HandJoint::WVR_HandJoint_Middle_Joint1,
Middle_Joint2 = 13,//WVR_HandJoint::WVR_HandJoint_Middle_Joint2,
Middle_Joint3 = 14,//WVR_HandJoint::WVR_HandJoint_Middle_Joint3, // 15
Middle_Tip = 15,//WVR_HandJoint::WVR_HandJoint_Middle_Tip,
Ring_Joint0 = 16,//WVR_HandJoint::WVR_HandJoint_Ring_Joint0,
Ring_Joint1 = 17,//WVR_HandJoint::WVR_HandJoint_Ring_Joint1,
Ring_Joint2 = 18,//WVR_HandJoint::WVR_HandJoint_Ring_Joint2,
Ring_Joint3 = 19,//WVR_HandJoint::WVR_HandJoint_Ring_Joint3, // 20
Ring_Tip = 20,//WVR_HandJoint::WVR_HandJoint_Ring_Tip,
Pinky_Joint0 = 21,//WVR_HandJoint::WVR_HandJoint_Pinky_Joint0,
Pinky_Joint1 = 22,//WVR_HandJoint::WVR_HandJoint_Pinky_Joint1,
Pinky_Joint2 = 23,//WVR_HandJoint::WVR_HandJoint_Pinky_Joint2,
Pinky_Joint3 = 24,//WVR_HandJoint::WVR_HandJoint_Pinky_Joint3, // 25
Pinky_Tip = 25,//WVR_HandJoint::WVR_HandJoint_Pinky_Tip,
};
EWaveVRHandGestureStatus¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRHandGestureStatus : uint8
{
// Initial, can call Start API in this state.
NOT_START,
START_FAILURE,
// Processing, should NOT call API in this state.
STARTING,
STOPING,
// Running, can call Stop API in this state.
AVAILABLE,
// Do nothing.
UNSUPPORT
};
EWaveVRHandTrackingStatus¶
UENUM(BlueprintType, Category = "WaveVR|Hand")
enum class EWaveVRHandTrackingStatus : uint8
{
// Initial, can call Start API in this state.
NOT_START,
START_FAILURE,
// Processing, should NOT call API in this state.
STARTING,
STOPING,
// Running, can call Stop API in this state.
AVAILABLE,
// Do nothing.
UNSUPPORT
};
Public Methods¶
GetDevicePose¶
To get the position and rotation of a device.
bool UWaveVRBlueprintFunctionLibrary::GetDevicePose(FVector& OutPosition, FRotator& OutOrientation, EWVR_DeviceType Type = EWVR_DeviceType::DeviceType_HMD)
SetPosePredictEnabled¶
To enable or disable position and rotation prediction of the device. HMD always apply rotation prediction and cannot be disabled. HMD position prediction and controller pose prediction are disabled by default.
void UWaveVRBlueprintFunctionLibrary::SetPosePredictEnabled(EWVR_DeviceType Type, bool enabled_position_predict, bool enabled_rotation_predict)
GetDeviceMatrix¶
To get the pose matrix of the device in view point with the specified type. Data are in left hand rules and positive Z is backward.
FMatrix UWaveVRBlueprintFunctionLibrary::GetDeviceMatrix(EWVR_DeviceType type = EWVR_DeviceType::DeviceType_Controller_Right)
GetDeviceVelocity¶
To get the velocity of a device. The data is in left hand Z-up coordinate.
FVector UWaveVRBlueprintFunctionLibrary::GetDeviceVelocity(EWVR_DeviceType type = EWVR_DeviceType::DeviceType_Controller_Right)
GetDeviceAngularVelocity¶
To get the angular velocity of a device. The data is in left hand Z-up coordinate.
FVector UWaveVRBlueprintFunctionLibrary::GetDeviceAngularVelocity(EWVR_DeviceType type = EWVR_DeviceType::DeviceType_Controller_Right)
IsDeviceConnected¶
To check if a device is connected.
bool UWaveVRBlueprintFunctionLibrary::IsDeviceConnected(EWVR_DeviceType Type)
IsDevicePoseValid¶
To check if a device has valid poses.
bool UWaveVRBlueprintFunctionLibrary::IsDevicePoseValid(EWVR_DeviceType Type)
GetSupportedNumOfDoF¶
To get the supported degree of freedom of a device.
EWVR_DOF UWaveVRBlueprintFunctionLibrary::GetSupportedNumOfDoF(EWVR_DeviceType Type)
SetTrackingHMDPosition¶
The position of HMD is (0,0,0) if IsTrackingPosition is false.
void UWaveVRBlueprintFunctionLibrary::SetTrackingHMDPosition(bool IsTrackingPosition)
IsTrackingHMDPosition¶
To get the latest status of calling SetTrackingHMDPosition.
bool UWaveVRBlueprintFunctionLibrary::IsTrackingHMDPosition()
SetTrackingHMDRotation¶
The rotation of HMD is (0,0,0) if IsTrackingRotation is false.
void UWaveVRBlueprintFunctionLibrary::SetTrackingHMDRotation(bool IsTrackingRotation)
SetTrackingOrigin3Dof¶
Set the HMD and controllers to rotation-only. Calling SetTrackingOrigin will cancel this effect.
void UWaveVRBlueprintFunctionLibrary::SetTrackingOrigin3Dof()
IsInputFocusCapturedBySystem¶
To check the application runs in foreground or background, false for foreground.
bool UWaveVRBlueprintFunctionLibrary::IsInputFocusCapturedBySystem()
InAppRecenter¶
To re-center the HMD position. Please refer to ERecenterType for the re-center type.
void UWaveVRBlueprintFunctionLibrary::InAppRecenter(ERecenterType type)
IsRenderFoveationSupport¶
To check whether the device supports the foveated render feature or not.
bool UWaveVRBlueprintFunctionLibrary::IsRenderFoveationSupport()
IsRenderFoveationEnabled¶
To check whether the foveated render feature is enabled or not.
bool UWaveVRBlueprintFunctionLibrary::IsRenderFoveationEnabled()
SetFoveationMode¶
To set foveated render mode.
void UWaveVRBlueprintFunctionLibrary::SetFoveationMode(EWVR_FoveationMode Mode = EWVR_FoveationMode::Default)
SetFoveationParams¶
To configure the foveated render feature.
EEye {LEFT, Right}
- Which eye the parameter will be applied.
Focal_X/Focal_Y
- The X/Y coordinate of the assigned eye. The original point (0,0) resides on the center of the eye. The domain value is between {-1, 1}.
FOV
- Represents the angle of the clear region.
EWVR_PeripheralQuality {Low, Medium, High}
- Represents the resolution of the peripheral region.
Note
Please make sure you disable AdaptiveQuality or enable AdaptiveQuality with Customization mode and deselect AutoFoveation because both AdaptiveQuality’s Quality/Performance oriented will enable AutoFoveation which will overwrite Foveated Rendering effects.
void UWaveVRBlueprintFunctionLibrary::SetFoveationParams(EEye Eye = EEye::LEFT, float Focal_X = 0.0f, float Focal_Y = 0.0f, float FOV = 90.0f, EWVR_PeripheralQuality PeripheralQuality = EWVR_PeripheralQuality::High)
GetFoveationParams¶
To get the foveated render parameter.
EEye {LEFT, Right}
- Which eye the parameter will be applied.
Focal_X/Focal_Y
- The X/Y coordinate of the assigned eye. The original point (0,0) resides on the center of the eye. The domain value is between {-1, 1}.
FOV
- Represents the angle of the clear region.
EWVR_PeripheralQuality {Low, Medium, High}
- Represents the resolution of the peripheral region.
Note
Please make sure you disable AdaptiveQuality or enable AdaptiveQuality with Customization mode and deselect AutoFoveation because both AdaptiveQuality’s Quality/Performance oriented will enable AutoFoveation which will overwrite Foveated Rendering effects.
void UWaveVRBlueprintFunctionLibrary::GetFoveationParams(EEye Eye, float& Focal_X, float& Focal_Y, float& FOV, EWVR_PeripheralQuality &PeripheralQuality)
IsAdaptiveQualityEnabled¶
To check if the AdaptiveQuality feature is enabled.
bool UWaveVRBlueprintFunctionLibrary::IsAdaptiveQualityEnabled()
EnableAdaptiveQuality_K2¶
To enable or disable the AdaptiveQuality feature with events sent and/or auto adjust foveation. The AutoFoveation will overwrite foveation mode. Warning: Please make sure you don’t need to use Foveated Rendering feature manually because both Quality/Performance oriented will overwrite Foveated Rendering effects.
bool UWaveVRBlueprintFunctionLibrary::EnableAdaptiveQuality_K2(bool Enable, EAdaptiveQualityMode Mode, bool SendQualityEvent, bool AutoFoveation)
SetPoseSimulationOption¶
To set the pose simulation type of the rotation-only controller. The simulation means the controller will use the arm model fake pose.
void UWaveVRBlueprintFunctionLibrary::SetPoseSimulationOption(SimulatePosition Option = SimulatePosition::WhenNoPosition)
SetFollowHead¶
To let the rotation-only controller which uses the simulation pose follow the head’s movement.
void UWaveVRBlueprintFunctionLibrary::SetFollowHead(bool follow = false)
getDeviceBatteryPercentage¶
Retrieves the connected device’s battery percentage.
float UWaveVRBlueprintFunctionLibrary::getDeviceBatteryPercentage(EWVR_DeviceType type)
GetRenderModelName¶
Retrieves the connected device’s render model name.
FString UWaveVRBlueprintFunctionLibrary::GetRenderModelName(EWVR_Hand hand)
GetRenderTargetSize¶
Retrieves the HMD’s eye buffer Resolution size.
bool UWaveVRBlueprintFunctionLibrary::GetRenderTargetSize(FIntPoint& OutSize)
IsLeftHandedMode¶
To check if the VR environment is left-handed mode.
bool UWaveVRBlueprintFunctionLibrary::IsLeftHandedMode()
GetDeviceErrorState¶
Retrieves the current states of device errors from the specified device.
Refer to System event for receiving event number 2009 first.
bool UWaveVRBlueprintFunctionLibrary::GetDeviceErrorState(EWVR_DeviceType Device, EWVR_DeviceErrorStatus Error)
EnableNeckModel¶
To enable or disable the neck model of a rotation-only head mount device. If enabled, the HMD will apply the neck model fake pose.
void UWaveVRBlueprintFunctionLibrary::EnableNeckModel(bool enable)
GetHoveredWidgetSeqId¶
To find the children of ParentWidget. The children widgets will be stored in ChildWidgets.
int UWaveVRBlueprintFunctionLibrary::GetHoveredWidgetSeqId(UUserWidget* ParentWidget, TArray<UWidget*>& ChildWidgets)
GetTexture2DFromImageFile¶
To retrieve texture2D image from a image file.
UTexture2D* UWaveVRBlueprintFunctionLibrary::GetTexture2DFromImageFile(FString imageFileName, FString imagePath, EUTex2DFmtType type)
GetWaveVRRuntimeVersion¶
Retrieves the API level of WaveVR SDK, e.g. SDK4.0 = API Level 6.
int UWaveVRBlueprintFunctionLibrary::GetWaveVRRuntimeVersion()
GetInputMappingPair¶
Retrieves the mapping key of a device button. E.g. Dominant Touchpad can be mapping from Left controller Thumbstick button.
EWVR_InputId UWaveVRBlueprintFunctionLibrary::GetInputMappingPair(EWVR_DeviceType type, EWVR_InputId button)
GetInputButtonState¶
To check if a device button is pressed.
bool UWaveVRBlueprintFunctionLibrary::GetInputButtonState(EWVR_DeviceType type, EWVR_InputId id)
GetInputTouchState¶
To check if a device button is touched.
bool UWaveVRBlueprintFunctionLibrary::GetInputTouchState(EWVR_DeviceType type, EWVR_InputId id)
IsButtonPressed¶
To check if a device button is pressed.
bool UWaveVRBlueprintFunctionLibrary::IsButtonPressed(EWVR_DeviceType type, EWVR_InputId id)
IsButtonTouched¶
To check if a device button is pressed.
bool UWaveVRBlueprintFunctionLibrary::IsButtonTouched(EWVR_DeviceType type, EWVR_TouchId id)
SetSplashParam¶
To configure the splash feature.
InSplashTexture
- The desired Texture which will be shown.
BackGroundColor
- The background color while showing a background removal texture (texture which has alpha channel).
ScaleFactor
- The amplification of the desired texture size, 1 is the original size.
Shift
- The shift offset by pixel applied to the texture in screen space. (0,0) means put the texture in the center.
EnableAutoLoading
- To show the splash texture while switching between maps or not.
void UWaveVRBlueprintFunctionLibrary::SetSplashParam(UTexture2D* InSplashTexture, FLinearColor BackGroundColor, float ScaleFactor, FVector2D Shift, bool EnableAutoLoading)
ShowSplashScreen¶
Manually force showing the splash texture.
void UWaveVRBlueprintFunctionLibrary::ShowSplashScreen()
HideSplashScreen¶
Manually force hding the splash texture.
void UWaveVRBlueprintFunctionLibrary::HideSplashScreen()
IsDirectPreview¶
Check if Direct Preview is running.
bool UWaveVRBlueprintFunctionLibrary::IsDirectPreview()
ScreenshotMode¶
To set the screenshot mode.
Please refer to the SDK documents for detailed information.
bool UWaveVRBlueprintFunctionLibrary::ScreenshotMode(EScreenshotMode ScreenshotMode)
GetScreenshotFileInfo¶
To retrieve the file name and the saved path of the screenshot.
void UWaveVRBlueprintFunctionLibrary::GetScreenshotFileInfo(FString &ImageFileName, FString &ImagePath)
SimulateCPULoading¶
This blueprint function is only used for debug. The unit of gameThreadLoading and renderThreadLoading are microsecond. And the values are both limited in 100 milliseconds. Set them to simulate CPU loadings on both thread.
void UWaveVRBlueprintFunctionLibrary::SimulateCPULoading(int gameThreadLoading, int renderThreadLoading)
IsLateUpdateEnabled¶
LateUpdate is default enabled. Use this function to check the status of LateUdate feature.
bool UWaveVRBlueprintFunctionLibrary::IsLateUpdateEnabled()
SetControllerPoseMode¶
To set up the controller pose mode.
Refer to the Controller Pose Mode for detailed information.
bool UWaveVRBlueprintFunctionLibrary::SetControllerPoseMode(EWVR_Hand Type, EWVR_ControllerPoseMode Mode)
GetControllerPoseMode¶
To retrieve current controller pose mode.
bool UWaveVRBlueprintFunctionLibrary::GetControllerPoseMode(EWVR_Hand Type, EWVR_ControllerPoseMode &OutMode)
GetControllerPoseModeOffset¶
To retrieve the controller offset of a pose mode.
bool UWaveVRBlueprintFunctionLibrary::GetControllerPoseModeOffset(EWVR_Hand Type, EWVR_ControllerPoseMode Mode, FTransform& OutTransform)
GetWorldToMetersScale¶
Retrieves the actual Unreal engine world scale. E.g. 1 : 1.01 meter.
float UWaveVRBlueprintFunctionLibrary::GetWorldToMetersScale()
SetFocusController¶
To specify the controller used to interact with objects.
void UWaveVRBlueprintFunctionLibrary::SetFocusController(EWVR_DeviceType focusedController)
GetFocusController¶
Retrieves the controller type used to interact with objects.
EWVR_DeviceType UWaveVRBlueprintFunctionLibrary::GetFocusController()
GetInteractionMode¶
Retrieves current interaction mode.
Refer to Interaction about the interaction mode information.
EWVRInteractionMode UWaveVRBlueprintFunctionLibrary::GetInteractionMode()
SetGazeTriggerType¶
To set the gaze trigger type: Timer or Button or both.
The gaze trigger type is only useful when the system is Gaze interaction mode.
bool UWaveVRBlueprintFunctionLibrary::SetGazeTriggerType(EWVRGazeTriggerType type)
GetGazeTriggerType¶
To retrieve the current gaze trigger type.
EWVRGazeTriggerType UWaveVRBlueprintFunctionLibrary::GetGazeTriggerType()
GetControllerAxis¶
Retrieves a controller button’s axis.
FVector2D UWaveVRController::GetControllerAxis(EWVR_DeviceType device, EWVR_TouchId button_id)
IsWaveVRInputInitialized¶
To check if the WaveVRInput feature is available.
bool UWaveVRInputFunctionLibrary::IsWaveVRInputInitialized()
GetWaveVRInputDeviceOrientation¶
To retrieve a device’s orientation. Supports left-handed mode.
FRotator UWaveVRInputFunctionLibrary::GetWaveVRInputDeviceOrientation(EWVR_DeviceType device = EWVR_DeviceType::DeviceType_Controller_Right)
GetWaveVRInputDevicePosition¶
To retrieve a device’s position. Supports left-handed mode.
FVector UWaveVRInputFunctionLibrary::GetWaveVRInputDevicePosition(EWVR_DeviceType device = EWVR_DeviceType::DeviceType_Controller_Right)
GetWaveVRInputDevicePositionAndOrientation¶
To retrieve the position and orientation of a device. Supports left-handed mode.
bool UWaveVRInputFunctionLibrary::GetWaveVRInputDevicePositionAndOrientation(EWVR_DeviceType device, FVector& OutPosition, FRotator& OutOrientation)
GetWaveVRInputDeviceTrackingStatus¶
To check if a device is tracked. Supports left-handed mode.
ETrackingStatus UWaveVRInputFunctionLibrary::GetWaveVRInputDeviceTrackingStatus(EWVR_DeviceType device = EWVR_DeviceType::DeviceType_Controller_Right)
GetWaveVRInputDeviceVelocity¶
To retrieve a device’s velocity. Supports left-handed mode.
FVector UWaveVRInputFunctionLibrary::GetWaveVRInputDeviceVelocity(EWVR_DeviceType device = EWVR_DeviceType::DeviceType_Controller_Right)
GetWaveVRInputDeviceAngularVelocity¶
To retrieve a device’s angular velocity. Supports left-handed mode.
FVector UWaveVRInputFunctionLibrary::GetWaveVRInputDeviceAngularVelocity(EWVR_DeviceType device = EWVR_DeviceType::DeviceType_Controller_Right)
UseSimulationPose¶
To simulate the device position when the environment is 3DoF (rotation only).
void UWaveVRInputFunctionLibrary::UseSimulationPose(SimulatePosition simulation = SimulatePosition::WhenNoPosition)
EnableInputSimulator¶
Activates the Input Simulator in PIE mode.
void UWaveVRInputFunctionLibrary::EnableInputSimulator(UObject * WorldContextObject)
FollowHeadPosition¶
To make the rotation-only controller which uses the simulation pose follows the head’s movement.
void UWaveVRInputFunctionLibrary::FollowHeadPosition(bool follow)
UpdateUnitySimulationSettingsFromJson¶
PIE only. This API is used for pose simulation customization.
void UWaveVRInputFunctionLibrary::UpdateUnitySimulationSettingsFromJson(FVector HEADTOELBOW_OFFSET, FVector ELBOWTOWRIST_OFFSET, FVector WRISTTOCONTROLLER_OFFSET, FVector ELBOW_PITCH_OFFSET, float ELBOW_PITCH_ANGLE_MIN, float ELBOW_PITCH_ANGLE_MAX)
IsInputAvailable¶
To check if a device is available. Supports left-handed mode.
bool UWaveVRInputFunctionLibrary::IsInputAvailable(EWVR_DeviceType device = EWVR_DeviceType::DeviceType_Controller_Right)
IsInputPoseValid¶
To check if a device’s pose is valid. Supports left-handed mode.
bool UWaveVRInputFunctionLibrary::IsInputPoseValid(EWVR_DeviceType device = EWVR_DeviceType::DeviceType_Controller_Right)
IsInputButtonPressed¶
To check if a device’s button is pressed. Supports left-handed mode.
bool UWaveVRInputFunctionLibrary::IsInputButtonPressed(EWVR_DeviceType device, EWVR_InputId button_id)
IsInputButtonTouched¶
To check if a device’s button is touched. Supports left-handed mode.
bool UWaveVRInputFunctionLibrary::IsInputButtonTouched(EWVR_DeviceType device, EWVR_TouchId button_id)
GetInputButtonAxis¶
To retrieve a button’s axis. Supports left-handed mode.
FVector2D UWaveVRInputFunctionLibrary::GetInputButtonAxis(EWVR_DeviceType device, EWVR_TouchId button_id)
TriggerHapticPulse¶
To vibrate the specified device in a duration of microseconds. Supports left-handed mode.
void UWaveVRInputFunctionLibrary::TriggerHapticPulse(EWVR_DeviceType device, int32 duration_ms)
GetInputMappingPair¶
To retrieve a button’s mapping information. E.g. Right thumbstick button is mapping to dominant touchpad key event. Supports left-handed mode.
EWVR_InputId UWaveVRInputFunctionLibrary::GetInputMappingPair(EWVR_DeviceType type, EWVR_InputId button)
GetDeviceBatteryPercentage¶
To retrieve a device’s battery percentage. Supports left-handed mode.
float UWaveVRInputFunctionLibrary::GetDeviceBatteryPercentage(EWVR_DeviceType type)
IsButtonAvailable¶
To check if a device’s button is available. Supports left-handed mode.
bool UWaveVRInputFunctionLibrary::IsButtonAvailable(EWVR_DeviceType type, EWVR_InputId button)
StartHandGesture¶
To enable the Hand Gesture component.
Hand Gesture is not supported in VIVE Wave™ plugin v4.1.
void UWaveVRHandBPLibrary::StartHandGesture()
StopHandGesture¶
To disable the Hand Gesture component.
Hand Gesture is not supported in VIVE Wave™ plugin v4.1.
void UWaveVRHandBPLibrary::StopHandGesture()
IsHandGestureAvailable¶
To check if the Hand Gesture component is available.
Hand Gesture is not supported in VIVE Wave™ plugin v4.1.
bool UWaveVRHandBPLibrary::IsHandGestureAvailable()
GetStaticGestureType¶
If the Hand Gesture component is available, this API is used to retrieve current static gesture type.
Hand Gesture is not supported in VIVE Wave™ plugin v4.1.
EWaveVRGestureType UWaveVRHandBPLibrary::GetStaticGestureType(EWaveVRHandType hand)
GetHandGestureStatus¶
To check current Hand Gesture status.
Hand Gesture is not supported in VIVE Wave™ plugin v4.1.
EWaveVRHandGestureStatus GetHandGestureStatus()
StartHandTracking¶
Note
Refer to Hand about the detailed information of Hand Tracking.
To enable the Hand Tracking component.
void StartHandTracking(EWaveVRTrackerType tracker)
Note
Only the natural hand tracker is supported currently.
StopHandTracking¶
To disable the Hand Tracking component.
void StopHandTracking(EWaveVRTrackerType tracker)
IsHandTrackingAvailable¶
To check if the Hand Tracking component is available.
bool IsHandTrackingAvailable(EWaveVRTrackerType tracker)
GetHandTrackingStatus¶
To check current Hand Tracking status.
EWaveVRHandTrackingStatus GetHandTrackingStatus(EWaveVRTrackerType tracker)
GetHandJointPose¶
If the Hand Tracking is available, this API is used to retrieve the left or right hand joint poses of the natural or electronic tracker.
bool GetHandJointPose(EWaveVRTrackerType tracker, EWaveVRHandType hand, EWaveVRHandJoint joint, FVector& OutPosition, FRotator& OutRotation)
The hand joints are defined as below photo:
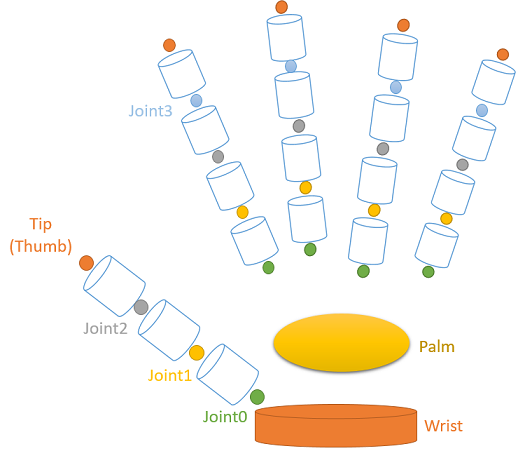
GetSingleHandJointPose¶
If the Hand Tracking is available, this API is used to retrieve the left or right hand joint poses of the natural or electronic tracker.
bool GetSingleHandJointPose(EWaveVRTrackerType tracker, EWaveVRHandType hand, EWaveVRHandJoint joint, FVector& OutPosition, FQuat& OutRotation)
GetAllHandJointPoses¶
If the Hand Tracking is available, this API is used to retrieve all left or right hand joint poses of the natural or electronic tracker.
bool GetAllHandJointPoses(EWaveVRTrackerType tracker, EWaveVRHandType hand, TArray<FVector>& OutPositions, TArray<FQuat>& OutRotations)
GetHandConfidence¶
To get the hand confidence (0~1), 1 means the most accurate.
float GetHandConfidence(EWaveVRTrackerType tracker, EWaveVRHandType hand)
GetHandPinchStrength¶
To get the hand pinch strength (0~1), 1 means the thumb and index finger tip are touching.
float GetHandPinchStrength(EWaveVRTrackerType tracker, EWaveVRHandType hand)
GetHandPinchOrigin¶
To get the hand pinch origin location in the world space.
Refer to below photo about the pinch origin and direction.
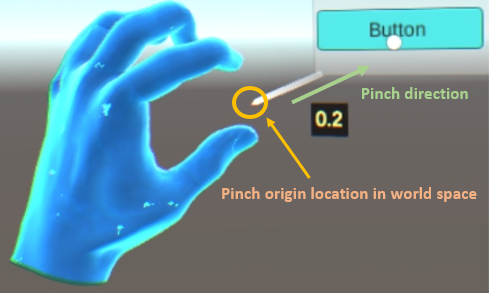
FVector GetHandPinchOrigin(EWaveVRTrackerType tracker, EWaveVRHandType hand)
GetHandPinchDirection¶
To get the hand pinch direction in the world space.
FVector GetHandPinchDirection(EWaveVRTrackerType tracker, EWaveVRHandType hand)