Tracker¶
Introduction¶
VIVE Wave™ plugin provides Blueprint APIs for miscellaneous information of Tracker. VIVE Tracker products contain Wrist Tracker and Ultimate Tracker
Blueprint Types¶
The Tracker interface uses blueprint types as below.
EWaveVRTrackerId¶
UENUM(BlueprintType, Category = "WaveVR|Tracker")
enum class EWaveVRTrackerId : uint8
{
Tracker0 = 0, //WVR_TrackerId.WVR_TrackerId_0
Tracker1 = 1, //WVR_TrackerId.WVR_TrackerId_1
Tracker2 = 2, //WVR_TrackerId.WVR_TrackerId_2,
Tracker3 = 3, //WVR_TrackerId.WVR_TrackerId_3,
Tracker4 = 4, //WVR_TrackerId.WVR_TrackerId_4,
Tracker5 = 5, //WVR_TrackerId.WVR_TrackerId_5,
Tracker6 = 6, //WVR_TrackerId.WVR_TrackerId_6,
Tracker7 = 7, //WVR_TrackerId.WVR_TrackerId_7,
Tracker8 = 8, //WVR_TrackerId.WVR_TrackerId_8,
Tracker9 = 9, //WVR_TrackerId.WVR_TrackerId_9,
Tracker10 = 10, //WVR_TrackerId.WVR_TrackerId_10,
Tracker11 = 11, //WVR_TrackerId.WVR_TrackerId_11,
Tracker12 = 12, //WVR_TrackerId.WVR_TrackerId_12,
Tracker13 = 13, //WVR_TrackerId.WVR_TrackerId_13,
Tracker14 = 14, //WVR_TrackerId.WVR_TrackerId_14,
Tracker15 = 15, //WVR_TrackerId.WVR_TrackerId_15,
};
EWaveVRTrackerRole¶
UENUM(BlueprintType, Category = "WaveVR|Tracker")
enum class EWaveVRTrackerRole : uint8
{
Undefined = 0, // WVR_TrackerRole.WVR_TrackerRole_Undefined,
Standalone = 1, // WVR_TrackerRole.WVR_TrackerRole_Standalone,
Pair1_Right = 2, // WVR_TrackerRole.WVR_TrackerRole_Pair1_Right,
Pair1_Left = 3, // WVR_TrackerRole.WVR_TrackerRole_Pair1_Left,
Shoulder_Right = 32, // WVR_TrackerRole_Shoulder_Right
Upper_Arm_Right = 33, // WVR_TrackerRole_Upper_Arm_Right
Elbow_Right = 34, // WVR_TrackerRole_Elbow_Right
Forearm_Right = 35, // WVR_TrackerRole_Forearm_Right
Wrist_Right = 36, // WVR_TrackerRole_Wrist_Right
Hand_Right = 37, // WVR_TrackerRole_Hand_Right
Thigh_Right = 38, // WVR_TrackerRole_Thigh_Right
Knee_Right = 39, // WVR_TrackerRole_Knee_Right
Calf_Right = 40, // WVR_TrackerRole_Calf_Right
Ankle_Right = 41, // WVR_TrackerRole_Ankle_Right
Foot_Right = 42, // WVR_TrackerRole_Foot_Right
Shoulder_Left = 47, // WVR_TrackerRole_Shoulder_Left
Upper_Arm_Left = 48, // WVR_TrackerRole_Upper_Arm_Left
Elbow_Left = 49, // WVR_TrackerRole_Elbow_Left
Forearm_Left = 50, // WVR_TrackerRole_Forearm_Left
Wrist_Left = 51, // WVR_TrackerRole_Wrist_Left
Hand_Left = 52, // WVR_TrackerRole_Hand_Left
Thigh_Left = 53, // WVR_TrackerRole_Thigh_Left
Knee_Left = 54, // WVR_TrackerRole_Knee_Left
Calf_Left = 55, // WVR_TrackerRole_Calf_Left
Ankle_Left = 56, // WVR_TrackerRole_Ankle_Left
Foot_Left = 57, // WVR_TrackerRole_Foot_Left
Chest = 62, // WVR_TrackerRole_Chest
Waist = 63, // WVR_TrackerRole_Waist
Camera = 71, // WVR_TrackerRole_Camera
Keyboard = 72, // WVR_TrackerRole_Keyboard
};
EWaveVRTrackerButton¶
UENUM(BlueprintType, Category = "WaveVR|Tracker")
enum class EWaveVRTrackerButton : uint8
{
System = 0,//WVR_InputId.WVR_InputId_0
Menu = 1,//WVR_InputId.WVR_InputId_Alias1_Menu
Grip = 2,//WVR_InputId.WVR_InputId_Alias1_Grip
A = 10,//WVR_InputId.WVR_InputId_Alias1_A
B = 11,//WVR_InputId.WVR_InputId_Alias1_B
X = 12,//WVR_InputId.WVR_InputId_Alias1_X
Y = 13,//WVR_InputId.WVR_InputId_Alias1_Y
Touchpad = 16,//WVR_InputId.WVR_InputId_Alias1_Touchpad
Trigger = 17,//WVR_InputId.WVR_InputId_Alias1_Trigger
};
EWaveVRTrackerStatus¶
UENUM(BlueprintType, Category = "WaveVR|Tracker")
enum class EWaveVRTrackerStatus : uint8
{
// Initial, can call Start API in this state.
NOT_START,
START_FAILURE,
// Processing, should NOT call API in this state.
STARTING,
STOPING,
// Running, can call Stop API in this state.
AVAILABLE,
// Do nothing.
UNSUPPORT
};
EWVR_AnalogType¶
UENUM(BlueprintType, Category = "WaveVR")
enum class EWVR_AnalogType : uint8
{
None = 0,
XY = 1,
XOnly = 2,
};
EWVR_DOF¶
UENUM(BlueprintType, Category = "WaveVR")
enum class EWVR_DOF : uint8
{
DOF_3,
DOF_6,
DOF_SYSTEM
};
Blueprint Function Library¶
You can see the Tracker functions by typing “Wave Tracker” in the blueprint.
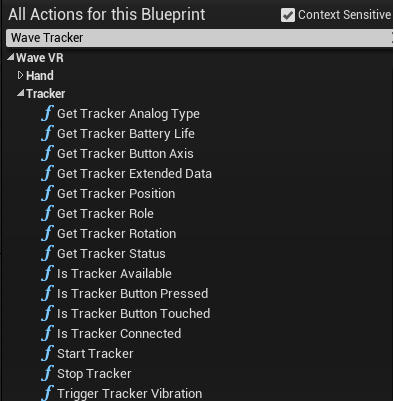
IsTrackerConnected¶
Checks if a Tracker is connected.
bool IsTrackerConnected(EWaveVRTrackerId trackerId)
GetTrackerRole¶
Retrieves a Tracker’s role. Refers to EWaveVRTrackerRole.
EWaveVRTrackerRole GetTrackerRole(EWaveVRTrackerId trackerId)
IsTrackerPoseValid¶
Checks if the tracker’s pose is valid.
bool IsTrackerPoseValid(EWaveVRTrackerId trackerId)
GetTrackerDegreeOfFreedom¶
Retrieves a Tracker’s degree of freedom. 3DoF for rotation only. 6DoF for position and rotation.
bool GetTrackerDegreeOfFreedom(EWaveVRTrackerId trackerId, EWVR_DOF & dof)
GetTrackerPosition¶
Retrieves a Tracker’s position.
bool GetTrackerPosition(EWaveVRTrackerId trackerId, FVector& outPosition) // returns true for valid position.
GetTrackerRotation¶
Retrieves a Tracker’s orientation.
bool GetTrackerRotation(EWaveVRTrackerId trackerId, FQuat& outOrientation) // returns true for valid rotation.
GetTrackerAnalogType¶
Retrieves a Tracker button’s analog type.
EWVR_AnalogType GetTrackerAnalogType(EWaveVRTrackerId trackerId, EWaveVRTrackerButton buttonId)
IsTrackerButtonPressed¶
Checks if a Tracker button is pressed.
bool IsTrackerButtonPressed(EWaveVRTrackerId trackerId, EWaveVRTrackerButton buttonId)
IsTrackerButtonTouched¶
Checks if a Tracker button is touched.
bool IsTrackerButtonTouched(EWaveVRTrackerId trackerId, EWaveVRTrackerButton buttonId)
GetTrackerButtonAxis¶
Retrieves a Tracker button’s axis.
FVector2D GetTrackerButtonAxis(EWaveVRTrackerId trackerId, EWaveVRTrackerButton buttonId)
GetTrackerBatteryLife¶
Retrieves a Tracker’s battery life in float (0~1) that 0 for 0% and 1 for 100%.
float GetTrackerBatteryLife(EWaveVRTrackerId trackerId)
TriggerTrackerVibration¶
Triggers a Tracker’s haptic pulse. Default duration is 0.5s. Set frequency to 0 to use system default value. Default amplitude (0~1) is 0.5f.
bool TriggerTrackerVibration(EWaveVRTrackerId trackerId, int durationMicroSec = 500000, int frequency = 0, float amplitude = 0.5f)
GetTrackerExtendedData¶
Retrieves a Tracker’s extended data.
TArray<int> GetTrackerExtendedData(EWaveVRTrackerId trackerId, int &validSize)
GetTrackerExtendedDataTimestamp¶
Retrieves a Tracker’s extended data with a timestamp.
TArray<int> GetTrackerExtendedDataTimestamp(EWaveVRTrackerId trackerId, int& validSize, int& timestamp)
GetTrackerDeviceName¶
Retrieves a Tracker’s device name.
For Wrist Tracker: the device name will be “Vive_Tracker_Wrist” or “Vive_Wrist_Tracker”.
For Ultimate Tracker: the device name will be “Vive_Tracker_OT”, “Vive_Self_Tracker” or “Vive_Ultimate_Tracker”.
bool GetTrackerDeviceName(EWaveVRTrackerId trackerId, FString& deviceName)
GetTrackerCallbackInfo¶
Retrieves a tracker’s callback string.
FString GetTrackerCallbackInfo(EWaveVRTrackerId trackerId)
RegisterTrackerInfoCallback¶
Registers the Tracker’s callback. You can retrieve the callback string after registered by using GetTrackerCallbackInfo.
bool RegisterTrackerInfoCallback(EWaveVRTrackerId trackerId)
UnregisterTrackerInfoCallback¶
Unregisters a Tracker’s callback.
bool UnregisterTrackerInfoCallback()
SetFocusedTracker¶
Specifies the tracker used to interact with objects.
void SetFocusedTracker(EWaveVRTrackerId focusedTracker)
GetFocusedTracker¶
Retrieves the tracker used to interact with objects. Returns true for a valid tracker.
bool GetFocusedTracker(EWaveVRTrackerId & trackerId)