Eye Expression¶
VIVE Wave™ plugin supports the Eye Expression feature. By following this guide you can retrieve the Eye Expression values as below illustrations.
- LEFT_BLINK: This blend shape influences blinking of the right eye. When this value goes higher, left eye approaches close.
- LEFT_WIDE: This blend shape keeps left eye wide and at that time LEFT_BLINK value is 0.
- RIGHT_BLINK: This blend shape influences blinking of the right eye. When this value goes higher, right eye approaches close.
- RIGHT_WIDE: This blend shape keeps right eye wide and at that time RIGHT_BLINK value is 0.
- LEFT_SQUEEZE: The blend shape closes eye tightly and at that time LEFT_BLINK value is 1.
- RIGHT_SQUEEZE: The blend shape closes eye tightly and at that time RIGHT_BLINK value is 1.
- LEFT_DOWN: This blendShape influences the muscles around the left eye, moving these muscles further downward with a higher value.
- RIGHT_DOWN: This blendShape influences the muscles around the right eye, moving these muscles further downward with a higher value.
- LEFT_OUT: This blendShape influences the muscles around the left eye, moving these muscles further leftward with a higher value.
- RIGHT_IN: This blendShape influences the muscles around the right eye, moving these muscles further leftward with a higher value.
- LEFT_IN: This blendShape influences the muscles around the left eye, moving these muscles further rightward with a higher value.
- RIGHT_OUT: This blendShape influences the muscles around the right eye, moving these muscles further rightward with a higher value.
- LEFT_UP: This blendShape influences the muscles around the left eye, moving these muscles further upward with a higher value.
- RIGHT_UP: This blendShape influences the muscles around the right eye, moving these muscles further upward with a higher value.
Enable Eye Expression¶
Before using the Eye Expression, you have to enable the feature from Project Settings > Plugins > Wave VR > Render > Enable Eye Tracking .
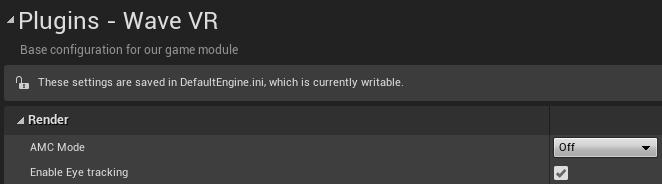
Blueprint Function Library¶
VIVE Wave™ plugin provides the Eye Expression interface as the following code which you can find at Plugins > WaveVR > Source > WaveVR > Public > EyeExpression.
UENUM(BlueprintType, Category = "WaveVR|Eye|Expression")
enum class EWaveVREyeExp : uint8
{
LEFT_BLINK = 0, // WVR_EyeExpression::WVR_EYEEXPRESSION_LEFT_BLINK
LEFT_WIDE = 1, // WVR_EyeExpression::WVR_EYEEXPRESSION_LEFT_WIDE
RIGHT_BLINK = 2, // WVR_EyeExpression::WVR_EYEEXPRESSION_RIGHT_BLINK
RIGHT_WIDE = 3, // WVR_EyeExpression::WVR_EYEEXPRESSION_RIGHT_WIDE
LEFT_SQUEEZE = 4, // WVR_EyeExpression::WVR_EYEEXPRESSION_LEFT_SQUEEZE
RIGHT_SQUEEZE = 5, // WVR_EyeExpression::WVR_EYEEXPRESSION_RIGHT_SQUEEZE
LEFT_DOWN = 6, // WVR_EyeExpression::WVR_EYEEXPRESSION_LEFT_DOWN
RIGHT_DOWN = 7, // WVR_EyeExpression::WVR_EYEEXPRESSION_RIGHT_DOWN
LEFT_OUT = 8, // WVR_EyeExpression::WVR_EYEEXPRESSION_LEFT_OUT
RIGHT_IN = 9, // WVR_EyeExpression::WVR_EYEEXPRESSION_RIGHT_IN
LEFT_IN = 10, // WVR_EyeExpression::WVR_EYEEXPRESSION_LEFT_IN
RIGHT_OUT = 11, // WVR_EyeExpression::WVR_EYEEXPRESSION_RIGHT_OUT
LEFT_UP = 12, // WVR_EyeExpression::WVR_EYEEXPRESSION_LEFT_UP
RIGHT_UP = 13, // WVR_EyeExpression::WVR_EYEEXPRESSION_RIGHT_UP
MAX, // WVR_EyeExpression::WVR_EYEEXPRESSION_MAX
};
UENUM(BlueprintType, Category = "WaveVR|Eye|Expression")
enum class EWaveVREyeExpStatus : uint8
{
// Initial, can call Start API in this state.
NOT_START,
START_FAILURE,
// Processing, should NOT call API in this state.
STARTING,
STOPING,
// Running, can call Stop API in this state.
AVAILABLE,
// Do nothing.
NO_SUPPORT
};
// To enable the Eye Expression component.
void StartEyeExp();
// To disable the Eye Expression component.
void StopEyeExp();
// Retrieves the Eye Expression component status.
EWaveVREyeExpStatus GetEyeExpStatus();
// Checks if the Eye Expression is available to use.
bool IsEyeExpAvailable();
// Retrieves an eye expression value.
float GetEyeExpValue(EWaveVREyeExp lipExp);
// Retrieves all eye expression data in a float array sorted in the order as EWaveVREyeExp emum.
bool GetEyeExpData(TArray<float>& OutValue);