Controller Buttons¶
Introduction¶
VIVE Wave™ supports buttons listed below:
- Menu
- Grip
- DPad Left
- DPad Up
- DPad Right
- DPad Down
- Volume Up
- Volume Down
- A
- B
- X
- Y
- Touchpad
- Trigger
- Thumbstick (Joystick)
- Parking
Note
We recommend to use the API
bool UWaveVRBlueprintFunctionLibrary::IsButtonAvailable
to check if a button is available.
Get Button State¶
Blueprint¶
In Blueprint, there are APIs can be used to get buttons’ press / touch states.
The buttons are define in WaveVRBlueprintFunctionLibrary.h
UENUM(BlueprintType)
enum class EWVR_InputId : uint8
{
NoUse = 0, //WVR_InputId::WVR_InputId_Alias1_System,
Menu = 1, //WVR_InputId::WVR_InputId_Alias1_Menu,
Grip = 2, //WVR_InputId::WVR_InputId_Alias1_Grip,
DPad_Left = 3, //WVR_InputId::WVR_InputId_Alias1_DPad_Left,
DPad_Up = 4, //WVR_InputId::WVR_InputId_Alias1_DPad_Up,
DPad_Right = 5, //WVR_InputId::WVR_InputId_Alias1_DPad_Right,
DPad_Down = 6, //WVR_InputId::WVR_InputId_Alias1_DPad_Down,
Volume_Up = 7, //WVR_InputId::WVR_InputId_Alias1_Volume_Up,
Volume_Down = 8, //WVR_InputId::WVR_InputId_Alias1_Volume_Down,
Bumper = 9, //WVR_InputId::WVR_InputId_Alias1_Bumper,
A = 10, //WVR_InputId::WVR_InputId_Alias1_A,
B = 11, //WVR_InputId::WVR_InputId_Alias1_B,
X = 12, //WVR_InputId::WVR_InputId_Alias1_X,
Y = 13, //WVR_InputId::WVR_InputId_Alias1_Y,
Back = 14, //WVR_InputId::WVR_InputId_Alias1_Back,
Enter = 15, //WVR_InputId::WVR_InputId_Alias1_Enter,
Touchpad = 16, //WVR_InputId::WVR_InputId_Alias1_Touchpad,
Trigger = 17, //WVR_InputId::WVR_InputId_Alias1_Trigger,
Thumbstick = 18, //WVR_InputId::WVR_InputId_Alias1_Thumbstick,
UENUM(BlueprintType)
enum class EWVR_TouchId : uint8
{
NoUse = 0, //WVR_InputId::WVR_InputId_Alias1_System,
Touchpad = 16, //WVR_InputId::WVR_InputId_Alias1_Touchpad,
Trigger = 17, //WVR_InputId::WVR_InputId_Alias1_Trigger,
Thumbstick = 18, //WVR_InputId::WVR_InputId_Alias1_Thumbstick,
Parking = 19, //WVR_InputId::WVR_InputId_Alias1_Parking
Right-click on the Blueprint Event Graph and type WaveVR to see APIs under subtitle:
- Input
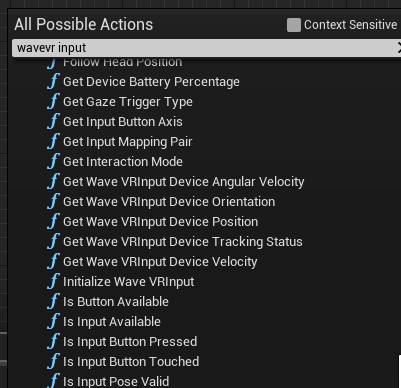
The API IsInputButtonPressed
, IsInputButtonTouched
and GetInputButtonAxis
of subtitle Input support left-handed mode.
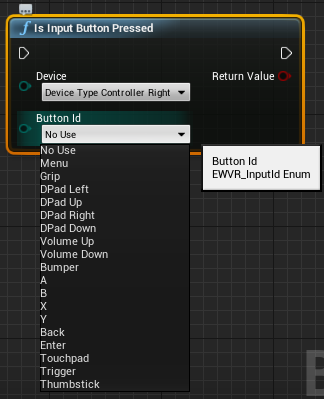
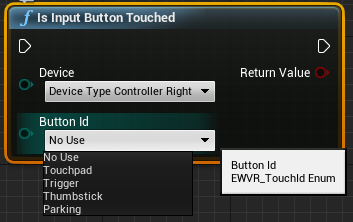
You can see the available Button Id
(NOT include Home/System/Power).
C++¶
In C++, you can use the sample code below to get the button_id
state of specified device
.
bool UWaveVRInputFunctionLibrary::IsInputButtonPressed(EWVR_DeviceType device, EWVR_InputId button_id)
bool UWaveVRInputFunctionLibrary::IsInputButtonTouched(EWVR_DeviceType device, EWVR_TouchId button_id)
FVector2D UWaveVRInputFunctionLibrary::GetInputButtonAxis(EWVR_DeviceType device, EWVR_TouchId button_id)
Unreal Input Plugin¶
Unreal provides the Engine Input .
In Settings -> Project Settings -> Engine/Input, you can define the Action & Axis Mapping via the Wave category to use the VIVE Wave™ Plugin buttons.
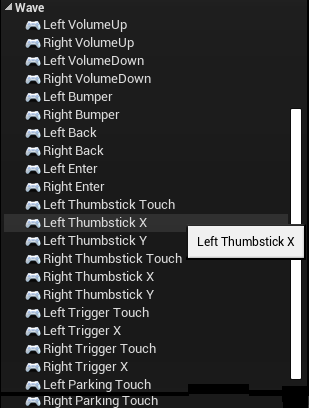
In C++ code (e.g. Pawn), you can listen to button’s state in SetupPlayerInputComponent
:
PlayerInputComponent->BindAction(<your defined action mapping>, IE_Pressed, this, <your function reference>);
PlayerInputComponent->BindAction(<your defined action mapping>, IE_Released, this, <your function reference>);
In Blueprint, you should Enable Input:
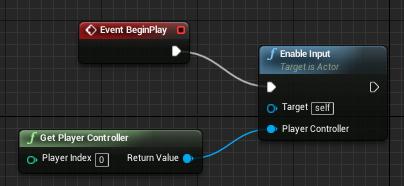
Add key(s) by right-click on the Blueprint Event Graph -> type Input Action Events
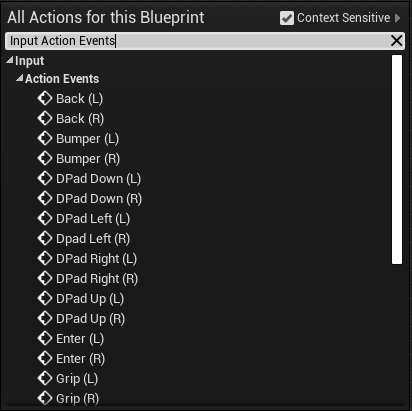