WVR_RenderFoveationMode¶
-
WVR_EXPORT WVR_Result WVR_RenderFoveationMode(WVR_FoveationMode mode)
Enable or disable foveated rendering or set the default mode for foveated rendering.
WVR_PreRenderEye must be called to apply foveated effect on a texture. This function is device dependent. WVR_IsRenderFoveationSupport must be called to check if the device supports foveated rendering. This function must be called after calling WVR_RenderInit.
- Version
- API Level 5
- Parameters
mode
: Change the foveated rendering mode.
- Return Value
WVR_Success
: Mode was successfully changed.others
: Failed to change the mode. See WVR_Result for more information.
Struct and enumeration¶
WVR_RenderFoveationParams_t is defined as below.
-
struct
WVR_RenderFoveationParams
¶ Foveation parameters.
Aggregate custom information of foveated rendering used in WVR_PreRenderEye.
Public Members
-
float
focalX
¶ focalX: The x coordinate of the focal point in normalized device coordinates. (-1.0 - 1.0)
-
float
focalY
¶ focalY: The y coordinate of the focal point in normalized device coordinates. (-1.0 - 1.0)
-
float
fovealFov
¶ fovealFov: The fov of the foveal.
-
WVR_PeripheralQuality
periQuality
¶ periQuality: The peripheral region quality of the foveated rendering.
-
float
-
enum
WVR_PeripheralQuality
¶ The peripheral region quality that is used in WVR_RenderFoveationParams_t.
Values:
-
WVR_PeripheralQuality_Low
= 0x0000¶ WVR_PeripheralQuality_Low: The peripheral region quality is low while power saved is high.
-
WVR_PeripheralQuality_Medium
= 0x0001¶ WVR_PeripheralQuality_Medium: The peripheral region quality is medium and power saved are average.
-
WVR_PeripheralQuality_High
= 0x0002¶ WVR_PeripheralQuality_High: The peripheral region quality is high while power saved is low.
-
-
enum
WVR_FoveationMode
¶ Foveated rendering mode.
Values:
-
WVR_FoveationMode_Disable
= 0x0000¶ WVR_FoveationMode_Disable: Disable foveated rendering.
-
WVR_FoveationMode_Enable
= 0x0001¶ WVR_FoveationMode_Enable: Enable foveated rendering and using WVR_SetFoveationConfig to set config.
-
WVR_FoveationMode_Default
= 0x0002¶ WVR_FoveationMode_Default: Fixed default config depending on the device. Using WVR_IsRenderFoveationDefaultOn and WVR_GetFoveationDefaultConfig to query details.
-
WVR_FoveationMode_Dynamic
= 0x0003¶ WVR_FoveationMode_Dynamic: Similar to default mode, but config will dynamically change due to HMD motion or eye tracking data while eye tracking module is enabled.
-
How to use¶
Foveated rendering is a technique used to reduce power consumption and improve performance. It reduce the resolution of the peripheral region while keeping the resolution of the foveal region clear and sharp. This correspond to two visions of the human visual system: foveal vision and peripheral vision. Foveal vision has the highest acuity while peripheral vision has lower acuity. When the resolution of images in the peripheral vision is reduced, people don’t notice the difference.
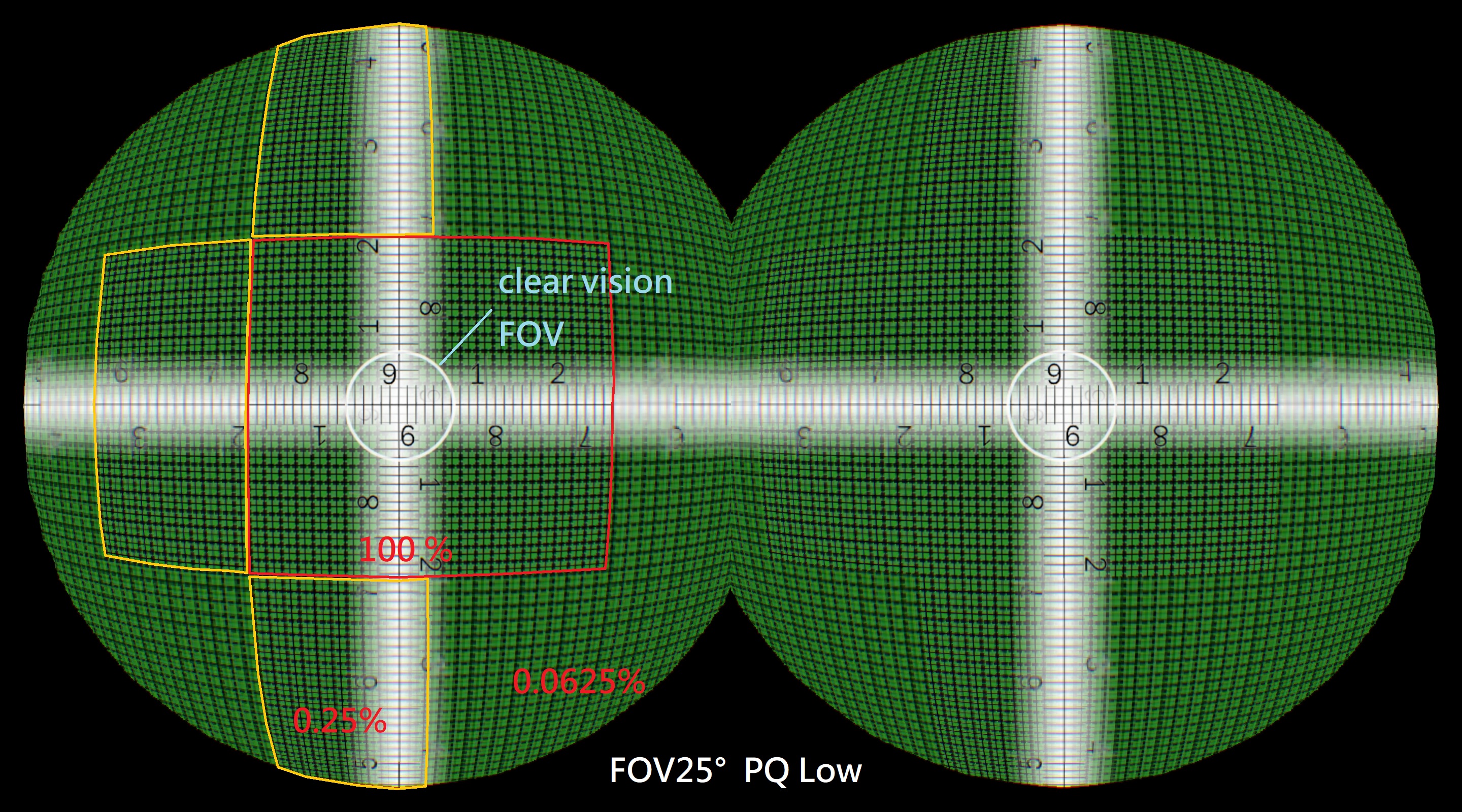
This sample image uses an extreme FOV setting to show obvious results. Settings are FOV25° and PeripheralQuality Low.
Foveation Mode¶
There are three available modes for WVR Foveation: Enable, Default, and Dynamic.
- WVR_FoveationMode_Enable : Enable mode gives the developer full control of the foveation configuration. This includes the focal offset, clear field of view, and periphery quality.
- WVR_FoveationMode_Default : Runtime will use a fixed default foveation configuration.
- WVR_FoveationMode_Dynamic : According to eye tracking module is available or not, the system will change foveation configuration dynamically:
- Eye tracking module is available and activating properly: the system will automatically adjust the focal offset and clear filed of view according to the eye tracking data to save GPU performance.
- If not, it will initialize as the same configuration as WVR_FoveationMode_Default, but it will dynamically change the field of view based on the motion of the HMD. As motion increases, the clear field of view will shrink.
Note¶
- For most of the cases, the default mode is a nice starting point. You can improve GPU performance with barely noticeable visual quality loss in periphery.
- If your rendering scene has no head locked objects, and the FPS can not reach refresh rate. You may try the dynamic mode for further performance improvement.
- Enabling foveated rendering feature incurs a fixed GPU performance cost, which can vary between 1% to 6% depending on the size of the eye buffer. When using a simple shader in the scene, the performance gain from saving resources might be lower than the fixed GPU performance cost, resulting in a performance drop. Therefore, it is advisable to enable or disable the feature based on your specific requirements and performance considerations.
- Eye tracking foveation behavior exhibits distinct variations across different SDK versions. Prior to SDK 6.1.0, the following characteristics apply:
- In WVR_FoveationMode_Default , eye tracking foveation is automatically enabled when the eye tracking module is available and functioning properly. If not, a fixed default configuration will be employed.
- WVR_FoveationMode_Dynamic always use head motion to adjust the field of view, and it will not change the focal offset.
Sample code¶
Default or Dynamic mode: Runtime handles most of the work.
#include <wvr/wvr_render.h>
// To enable foveated rendering
WVR_RenderFoveationMode(WVR_FoveationMode_Default); // Or WVR_FoveationMode_Dynamic
while(true) {
// Step 1 PreRenderEye. Runtime will set up texture parameters for foveated rendering.
// Left eye
int32_t IndexLeft = WVR_GetAvailableTextureIndex(mLeftEyeQ);
WVR_TextureParams_t eyeTexture = WVR_GetTexture(mLeftEyeQ, IndexLeft);
WVR_PreRenderEye(WVR_Eye_Left, &eyeTexture);
// Right eye
int32_t IndexRight = = WVR_GetAvailableTextureIndex(mRightEyeQ);
eyeTexture = WVR_GetTexture(mRightEyeQ, IndexRight);
WVR_PreRenderEye(WVR_Eye_Right, &eyeTexture);
// Step 2 Render scene
// Step 3 Submit texture
WVR_SubmitError e;
// Left eye
e = WVR_SubmitFrame(WVR_Eye_Left, &leftEyeTexture);
// Right eye
e = WVR_SubmitFrame(WVR_Eye_Right, &rightEyeTexture);
}
Enable(Custom) mode: Developers assign desired foveated rendering parameters.
#include <wvr/wvr_render.h>
// To enable foveated rendering
WVR_RenderFoveationMode(WVR_FoveationMode_Enable);
while(true) {
// Step 1 PreRenderEye. Runtime will set up texture parameters using the given foveated rendering parameters.
// Left eye
int32_t IndexLeft = WVR_GetAvailableTextureIndex(mLeftEyeQ);
WVR_TextureParams_t eyeTexture = WVR_GetTexture(mLeftEyeQ, IndexLeft);
{
WVR_RenderFoveationParams_t foveated;
foveated.focalX = foveated.focalY = 0.0f; // focal position
foveated.fovealFov = 30.0f; // 30 degrees
foveated.periQuality = static_cast<WVR_PeripheralQuality>(WVR_PeripheralQuality_High);
WVR_PreRenderEye(WVR_Eye_Left, &eyeTexture, &foveated);
}
// Right eye
int32_t IndexRight = = WVR_GetAvailableTextureIndex(mRightEyeQ);
eyeTexture = WVR_GetTexture(mRightEyeQ, IndexRight);
{
WVR_RenderFoveationParams_t foveated;
foveated.focalX = foveated.focalY = 0.0f; // focal position
foveated.fovealFov = 30.0f; // 30 degrees
foveated.periQuality = static_cast<WVR_PeripheralQuality>(WVR_PeripheralQuality_High);
WVR_PreRenderEye(WVR_Eye_Right, &eyeTexture, &foveated);
}
// Step 2 Render scene
// Step 3 Submit texture
WVR_SubmitError e;
// Left eye
e = WVR_SubmitFrame(WVR_Eye_Left, &leftEyeTexture);
// Right eye
e = WVR_SubmitFrame(WVR_Eye_Right, &rightEyeTexture);
}
Peripheral Region Quality Samples
Reminders
Things to remember for foveated rendering:
- Final rendering results may differ on different devices.
- Some devices do not support foveated rendering: Use WVR_IsRenderFoveationSupport to check if the device supports foveated rendering.
- If MSAA and foveated rendering are used at the same time. MSAA will affect the final rendering results of foveated rendering.
- Do not use tessellation, geometry, or compute shaders with foveated rendering.
- Periphery quality drop may be noticed while screen recording or miracasting.
Warning
The foveation setting here would be loss effect during AdaptiveQuality enabled with WVR_QualityStrategy_AutoFoveation strategy. It has be controlled by Adaptive Quality.