Dynamic Resolution¶
Introduction¶
Dynamic Resolution is a feature which helps adjust the image quality of the application according to events sent by AdaptiveQuality. Therefore, AdaptiveQuality must be enabled in order to use Dynamic Resolution. Adjustments to image quality are made by changing the Resolution Scale according to the event type received from AdaptiveQuality.
When the event type received is WVR_EventType_RecommendedQuality_Higher
, the resolution will be increased.
When the event type received is WVR_EventType_RecommendedQuality_Lower
, the resolution will be decreased.
To make sure that Resolution Scale will not decrease to a point where the application is unusable, Dynamic Resolution has a built-in functionality that helps determine the lower bound of Resolution Scale for different VR devices.
To use Dynamic Resolution in Wave Unity XR Plugin, it needs to be enabled first in WaveXRSettings . You can customize the global Dynamic Resolution settings in WaveXRSettings or put a Dynamic Resolution component in a GameObject in a scene to create a specific setting for that scene.
Since the component will spawn automatically using the global settings at the beginning of each scene, you do not need to manually put a Dynamic Resolution component in a GameObject in a scene unless you want to create custom settings for a scene.
Adaptive Quality Mode¶
With the introduction of Adaptive Quality Mode, the behavior of Dynamic Resolution will also change according to the Adaptive Quality Mode selected in WaveXRSettings.
Resolution Scale List Presets
When Quality Oriented Mode or Performance Oriented Mode is selected, Dynamic Resolution will apply different Resolution Scale List Presets accordingly and the global Dynamic Resolution settings cannot be override.
When Customization Mode is selected, you can customize the Resolution Scale List and override the global settings by creating custom settings for a scene.Resolution Scale Bounds
Each Adaptive Quality Mode uses different combinations of Upper and Lower Bounds of Resolution Scale as follow:
Adaptive Quality Mode Lower Bound Upper Bound Quality Oriented Mode 1 Provided by Wave Runtime Performance Oriented Mode Calculated by Dynamic Resolution 1 Customization Mode Calculated by Dynamic Resolution Provided by Wave Runtime
Resolution Scale¶
Resolution Scale is changed through Unity API XRSettings.eyeTextureResolutionScale.
The highest Resolution Scale reachable during runtime is defined as either the highest Resolution Scale value in the Resolution Scale List or the upper bound used by the selected Adaptive Quality Mode. The lower value between the two will be chosen.
The lowest Resolution Scale reachable during runtime is defined as either the lowest Resolution Scale value in the Resolution Scale List or the lower bound used by the selected Adaptive Quality Mode. The higher value between the two will be chosen.
Upper Bound provided by Wave Runtime¶
The Wave Runtime provides a Resolution Scale upper bound specific to each device. This allows image quality to be improved by increasing the Resolution Scale when there is headroom in system resources.
Lower Bound calculated by Dynamic Resolution¶
Dynamic Resolution calculates the lower bound for the Resolution Scale based on the readability of UI text elements. As the text size gets smaller, the lower bound for the Resolution Scale increases, as more rendered pixels (i.e. larger Render Texture size) is needed to make the text readable. The Text Size in Dynamic Resolution is measured in dmm (Distance-Independent Millimeters), where dmm is an angular unit. For example, 1 mm at 1 meter distance and 2 mm at 2 meter distance are both considered as 1 dmm.
To obtain the corresponding dmm from Unity UI Font Size, we must first convert Unity UI Font Size to real life units. At transform scale (1,1,1), the text height at Font Size 14 is 10 meters, so the coefficient to transform real World Space Height to Unity UI Font Size is 1.4. Since dmm uses millimeters for text height, we then convert the real World Space Height from meters to millimeters. Then, take the distance between the user (position of headset) and text (canvas for holding the UI text elements) and divide the text height with it. Therefore, to convert Unity UI Text Font size to dmm, use this formula:
((Unity Font Size x Transform Scale)/ 1.4) x 1000 / Intended Reading Distance = Text Size in dmm
For example, if Unity Font Size is 30, the Transform Scale of the text canvas is 0.001. If you want to make the text readable when the user is a meter away from the text canvas, the text size should be ((30 x 0.001)/1.4) x 1000 / 1 = 21.42857~ dmm. For simplicity, round up the value to 22 dmm and set it in the inspector.
Recommended Practice: Use text size of 20 dmm or larger. Text sizes of 20 dmm - 40 dmm are commonly used in other mediums such as webpages and are known to provide a relatively comfortable reading experience. While you can always manually set a lower bound in the list that is high enough to make text with text size smaller than 20 dmm readable, we do not recommend this from an application design standpoint.
Inspector¶
Override XR Settings
bool
If you wish to override the global Dynamic Resolution settings, set this field to true. You can then access the other parameters through the inspector, and those parameters will be applied to the current scene instead of the global settings.
Text Size
int
You can set the text size according to your application design. The default text size is 20 dmm, which is also the minimum recommended text size.
Resolution Scale List
List<float>
You need to define a list of Resolution Scale for Dynamic Resolution to make adjustments accordingly. In the list, value of index 0 maps to the highest quality level. The highest Resolution Scale value should be set here. Values of increasing index map to lower quality levels, and lower Resolution Scale values should be set in decreasing numerical order.
When Dynamic Resolution receives events of type
WVR_EventType_RecommendedQuality_Higher
, it will decrease the current index by 1 (one quality level higher) and use the Resolution Scale value with the new index from the list.When Dynamic Resolution receives events of type
WVR_EventType_RecommendedQuality_Lower
, it will increase the current index by 1 (one quality level lower) and use the Resolution Scale value with the new index from the list.Default Index
int
The Resolution Scale value at the Default Index is the initial value used before receiving any events from AdaptiveQuality.
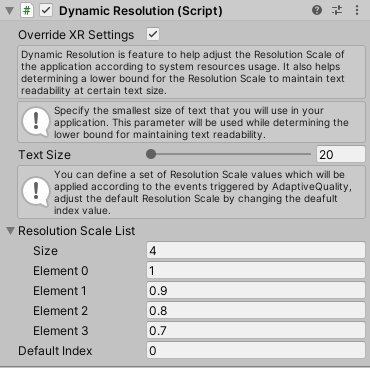
Dynamic Resolution Component with Override XR Settings enabled
Script¶
Public Types¶
enum AQEvent {
None,
ManualHigher,
ManualLower,
Higher,
Lower
};
None
means no events are received.ManualHigher
andManualLower
means that the change in Resolution Scale is triggered manually by using public member function.Higher
andLower
means that the received event was sent by AdaptiveQuality.
Properties¶
float CurrentScale { get; }
Get the mapped Resolution Scale value of the current index from the Resolution Scale List.
AQEvent CurrentAQEvent { get; }
Get the current received event sent by AdaptiveQuality or manual trigger.
Public Member Functions¶
void Higher()
Manually increase the quality instead of waiting for an event from AdaptiveQuality. It will decrease current index by 1.
void Lower()
Manually reduce the quality instead of waiting for an event from AdaptiveQuality. It will increase current index by 1.
void ResetResolutionScale()
Reset the current index to default index and update the Resolution Scale accordingly.