Input Module¶
Introduction¶
An input module holds the main logic that controls the behavior of the Event System. The module is used for:
- Handling input
- Managing event state
- Sending events to scene objects.
In Unity, go to Project Settings > Wave XR > Essence to import the Input Module package.
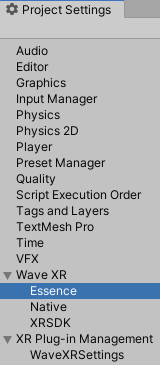

The imported content of the Input Module package is located in Assets/Wave/Essence/InputModule and provides three input modules: GazeInputModule, ControllerInputModule and HandInputModule.
If you met problems when using the Input Module, refer to Input Module Usage Notification.
Gaze Input¶
An Event System is a Unity UI component that can be added from Game Object > UI > Event System. To use the GazeInputModule, add the GazeInputModule to the EventSystem object.
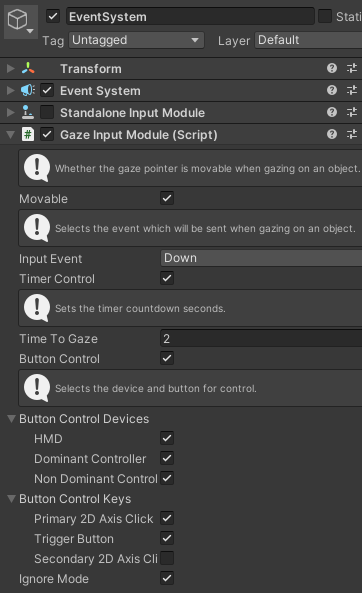
Movable
: Whether the gaze pointer moves when gazing on an object.
Input Event
: There are two events, Down and Submit, that can be sent when gazing on an object.
Timer Control
: Select to use a timer to trigger gaze events when gazing. Input the countdown duration in seconds in Time to Gaze
.
Button Control
: Select to use controller buttons to trigger gaze events. If selected, set Button Control Devices
and Button Control Keys
as well.
Ignore Mode
: The GazeInputModule can only be used in Gaze mode by default unless this value is set.
Controller Input¶
An Event System is a Unity UI component that can be added from Game Object > UI > Event System. To use the ControllerInputModule, add the ControllerInputModule to the EventSystem object.
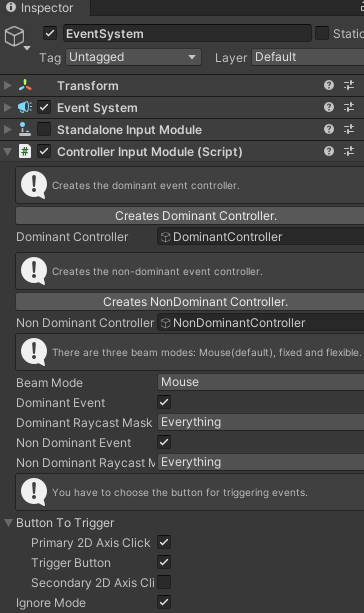
Creates Dominant Controller
: Click on the button to generate a dominant controller or specify a GameObject with the EventControllerSetter as the dominant controller.
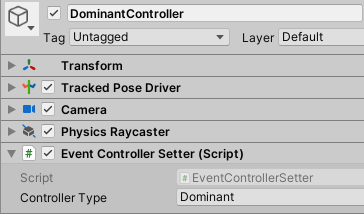
Creates NonDominant Controller
: Click on the button to generate a non-dominant controller or specify a GameObject with the EventControllerSetter
as the non-dominant controller.
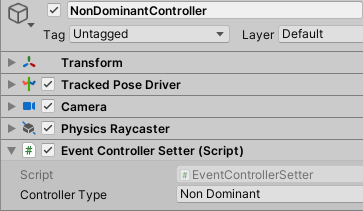
Beam Mode
: There are three modes for different beam types.
- Flexible: The pointer is located at the intersecting point where the controller raycast hits an object.
When the controller raycast is not hitting anything, the position of the pointer will change based on the controller rotation, while its distance from the controller will either be the default distance or the distance of the previously intersecting point.
![]()
- Fixed: The length of the controller beam is fixed and there is no controller pointer. There will be an intersecting point if the controller raycast hits an object.
- Mouse: The raycast from the eye (line of sight) goes through the pointer, which its position will change based on controller rotation. There will be an intersecting point if the line of sight hits an object.
Dominant Raycast Mask
and Non Dominant Raycast Mak
: Two controllers can be used in the ControllerInputModule: Dominant and Non-Dominant. Each controller has its own PhysicsRaycaster eventMask .
Note
By default, the Dominant type is equivalent to XRNode.RightHand and the Non-Dominant type is equivalent to XRNode.LeftHand
Button To Trigger
: Select which buttons are used to trigger events.
Fixed Beam Length
: Set the length of the beam when the Beam Mode
is set to Fixed.
Ignore Mode
: The ControllerInputModule can only be used in Controller mode by default unless this value is set.
Hand Input¶
An EventSystem is a Unity UI component that can be added from Game Object > UI > Event System. To use the HandInputModule, add the HandInputModule to the EventSystem object.
Note
The HandInputModule is usable only when the UnityXRHand is available.
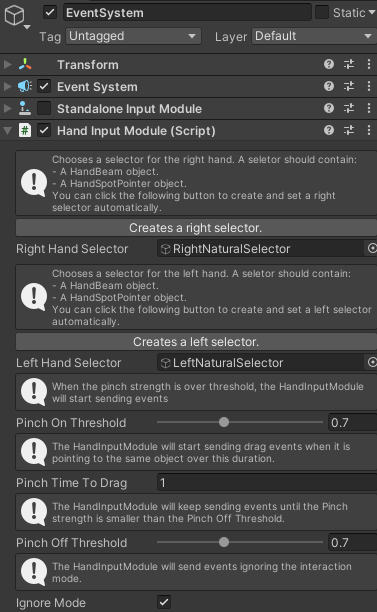
Create a right selector
: You can click on the button to generate a right selector or specify a GameObject with the HandBeam and HandSpotPointer as the selector.
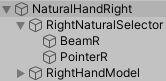
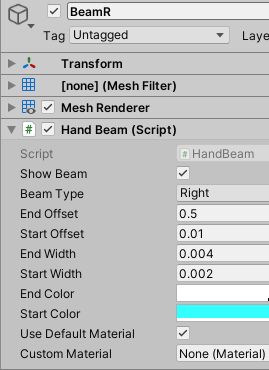
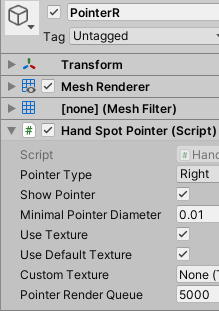
Create a left selector
: You can click on the button to generate a left selector or specify a GameObject with the HandBeam and HandSpotPointer as the selector.
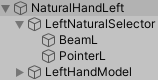
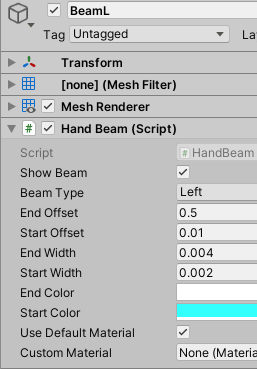
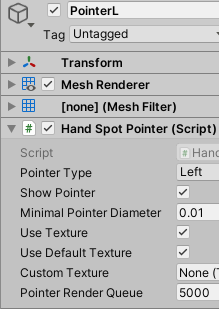
A hand with a selector looks like the below image.
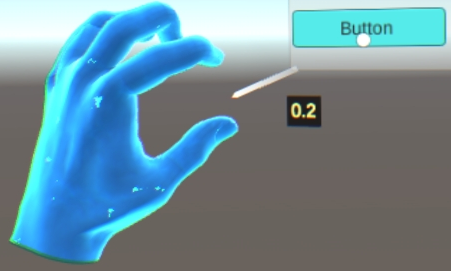
The HandInputModule applies the Pinch origin and direction to the selector.
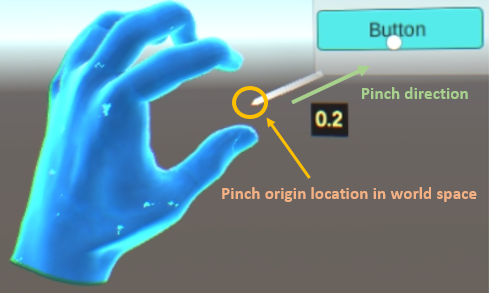
Get the Pinch origin and direction by using the following code:
Vector3 pinchOrigin = Vector3.zero, pinchDirection = Vector3.zero;
HandManager.Instance.GetPinchOrigin(ref pinchOrigin, HandManager.HandType.Left); // gets left pinch origin.
HandManager.Instance.GetPinchDirection(ref pinchDirection, HandManager.HandType.Left); // gets left pinch direction.
The Pinch gesture looks like:
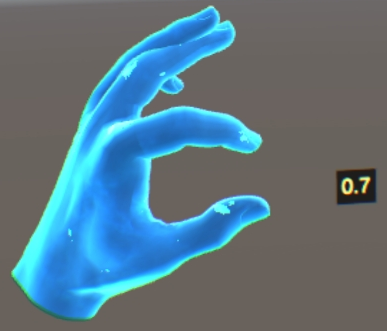
The yellow numerical value represents the Pinch gesture strength value.
Get the Pinch strength value by using the following code:
float pinchStrength = HandManager.Instance.GetPinchStrength(HandManager.HandType.Left); // gets left pinch strength.
The Pinch strength value is between 0 and 1, where 1 means that the thumb and the tip of the index finger are touching.
You have to set the threshold of the Pinch. When the Pinch strength value is bigger than the Pinch On Threshold
, the HandInputModule will start sending events.
The HandInputModule will keep sending events until the Pinch strength value is smaller than the Pinch Off Threshold
.
The HandInputModule will start sending drag events when it is pointing to the same object over Pinch Time To Drag
.
Ignore Mode
: The HandInputModule can only be used in Hand mode by default unless this value is set.